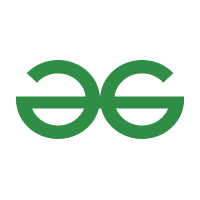
Sort String Characters: Fastest Methods for Alphabetical Order (C++, Python, Java)
Want to arrange the characters in a string alphabetically? This guide dives into efficient methods for sorting strings in various programming languages, going beyond the basics to achieve optimal performance. No more hunting for the right algorithm!
Why Sort Strings Alphabetically?
Sorting strings alphabetically has many practical applications:
- Data Organization: Easily arrange lists of names, items, or keywords for better readability.
- Search Optimization: Sorted data facilitates faster searching and retrieval.
- Anagram Detection: Determining if two strings are anagrams becomes straightforward after sorting.
- Lexicographical Order: Many algorithms rely on lexicographical order (alphabetical order) for processing text.
Naive Approach: Sorting with O(n Log n) Time Complexity
The simplest method involves using standard sorting algorithms like quicksort or mergesort. These algorithms generally have a time complexity of O(n log n), where n is the length of the string.
- Easy to Implement: Most languages provide built-in sorting functions.
- Not the most Efficient: For strings with a limited character set, better options exist.
Below are examples in various languages:
C++
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
int main() {
string s = "geeksforgeeks";
sort(s.begin(), s.end());
cout << s << endl;
return 0;
}
Java
Python
Efficient Approach: Counting Sort with O(n) Time Complexity
For strings containing only lowercase characters (a-z), we can achieve linear time complexity O(n) using a counting sort approach. This method leverages the limited character set to optimize sorting.
How Counting Sort Works for Strings
- Create a Count Array: Initialize an array of size 26 (for each lowercase letter) with all elements set to 0.
- Count Character Occurrences: Iterate through the input string, incrementing the corresponding count in the array for each character. For example, if the string contains 'a', increment
count['a' - 'a']
which iscount[0]
. - Rebuild the Sorted String: Iterate through the count array. For each character, append it to the result string the number of times it appears in the count.
- Linear Time Complexity: Offers significant performance improvement over comparison-based sorts for specific cases.
- Space Complexity: Requires extra space for the count array (constant size of 26 for lowercase letters).
C++
#include <iostream>
#include <string>
using namespace std;
const int MAX_CHAR = 26;
void sortString(string& s) {
int charCount[MAX_CHAR] = {0};
for (int i = 0; i < s.length(); i++) {
charCount[s[i] - 'a']++;
}
s = ""; // Clear the original string
for (int i = 0; i < MAX_CHAR; i++) {
for (int j = 0; j < charCount[i]; j++) {
s += (char)('a' + i);
}
}
}
int main() {
string s = "geeksforgeeks";
sortString(s);
cout << s << endl;
return 0;
}
Choosing the Right Approach
Approach | Time Complexity | Space Complexity | Best Use Case |
---|---|---|---|
Naive (sort()) | O(n log n) | O(1) | General-purpose sorting, unknown character set. |
Counting Sort | O(n) | O(1) | Strings with a limited, known character set (a-z). |
Consider the characteristics of your input strings when selecting a sorting method. If you know the input contains only lowercase letters, the counting sort approach provides optimal performance. Otherwise, use the naive approach for a general solution.