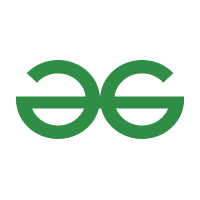
Mastering String Manipulation: How to Count Word Occurrences in Python and More
Do you need to analyze text, count specific words, or process large amounts of data? Learning how to efficiently count the occurrences of a word within a string is a fundamental skill for any programmer. This article will guide you through various methods, offering clear examples in multiple languages.
Why Count Word Occurrences?
Counting word occurrences is useful for several applications across different fields like:
- Data analysis: Identify frequently used topics or keywords.
- SEO optimization: Assess keyword density in web content.
- Natural language processing: Analyze text, find themes, and construct data.
- Content moderation: Discover abusive terms in user messages.
Method 1: Splitting the String
This method involves splitting the input string into smaller chunks and checking equality to find the number of times a certain word appears in a string.
- Split the string into an array of words.
- Iterate through the array.
- Increment a counter each time the target word is found.
Here's how it looks like in Python 3:
C++ Example:
#include <iostream>
#include <vector>
#include <string>
#include <cstring>
using namespace std;
int countOccurrences(char* str, string word) {
char* p;
vector<string> a;
p = strtok(str, " ");
while (p != NULL) {
a.push_back(p);
p = strtok(NULL, " ");
}
int c = 0;
for (int i = 0; i < a.size(); i++)
if (word == a[i])
c++;
return c;
}
int main() {
char str[] = "GeeksforGeeks A computer science portal for geeks ";
string word = "portal";
cout << countOccurrences(str, word);
return 0;
}
This approach has a time complexity of O(n) and needs O(n) auxiliary space.
Method 2: Leveraging Python's count()
Function
Python provides a built-in count()
function which simplifies the process. This solution uses the method to find the amount of times an element appears in a list.
- Split the string into a list of words.
- Employ the
count()
function to determine the number of occurrences of the target word.
Here's the Java equivalent:
Also with time complexity of O(n) and needs O(n) auxiliary space.
Method 3: Regular Expressions with re.findall
For more complex patterns or when dealing with variations of a word, regular expressions offer a robust solution. This method is great for counting word appearance, even with additional conditions.
- Import the
re
module. - Use
re.findall()
to find all occurrences of the word. - Return the length of this list.
C++ equivalent:
#include <iostream>
#include <string>
#include <regex>
using namespace std;
int count_word_occurrences2(const string& str, const string& word) {
regex regex_word(word);
sregex_iterator it(str.begin(), str.end(), regex_word);
sregex_iterator end;
int count = 0;
while (it != end) {
count++;
++it;
}
return count;
}
int main() {
string str = "GeeksforGeeks A computer science portal for geeks";
string word = "portal";
int count = count_word_occurrences2(str, word);
cout << "Occurrences of Word = " << count << " Time" << endl;
return 0;
}
Choosing the Right Approach
The best method depends on the specific requirements:
- For simple exact matches,
split()
andcount()
are efficient. - For complex criteria, regular expressions provide flexibility.
Counting word occurrences is a vital competence in programming and data analysis. By understanding these methods, you can process text effectively and gain valuable insights from your data!