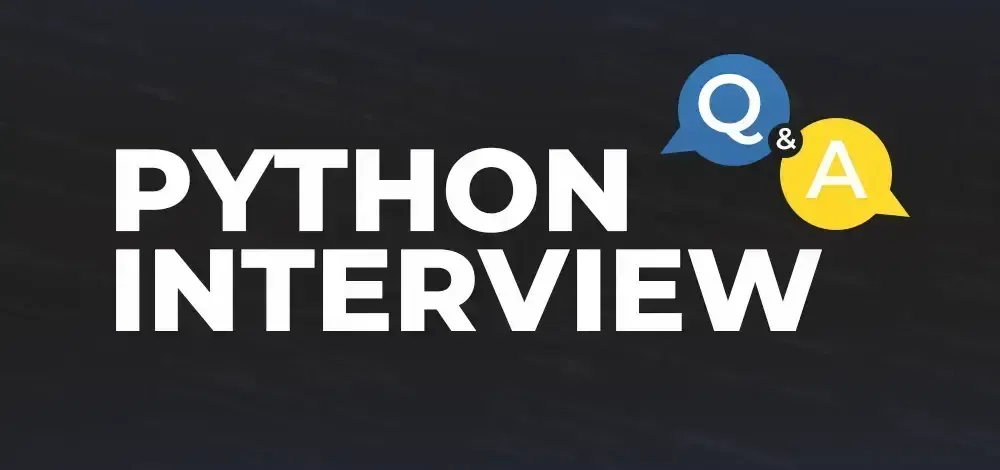
Python Interview Questions & Answers: Ace Your Coding Interview in 2024
Are you preparing for a Python coding interview? Getting hired as a Python developer at top companies like Netflix, Facebook, or Spotify requires more than just knowing the language. It demands mastering key concepts and demonstrating your ability to solve real-world problems.
This comprehensive guide covers the top 50 Python interview questions and answers to help you confidently navigate the interview process and impress your potential employers. Whether you're a fresher, intermediate, or advanced Python developer, we've got you covered.
Python Interview Questions for Freshers: Basic Concepts
Let's start with the fundamental concepts that every Python developer should know.
1. Compiled or Interpreted: Understanding Python's Execution
Is Python a compiled or interpreted language? The answer is both!
- Compilation: Python first compiles your source code into bytecode (
.pyc
files). - Interpretation: The Python Virtual Machine (PVM) then interprets this bytecode line by line.
Some implementations, like PyPy, use Just-In-Time (JIT) compilation for faster execution.
2. Dynamically Typed: What it Means for Python
What's a dynamically typed language?
- Statically typed languages (e.g., C++, Java) require you to declare the data type of variables.
- Dynamically typed languages (e.g., Python, JavaScript) determine the data type at runtime.
- Python's dynamic typing makes coding faster but can lead to runtime errors if not handled carefully.
3. Indentation Matters: Python's Unique Syntax Requirement
Is indentation required in Python? Absolutely!
- Indentation defines code blocks, replacing curly braces in other languages.
- Correct indentation is crucial for Python code to execute properly.
- It also promotes readability, making the code easier to understand and maintain.
4. Built-in Data Types: A Quick Overview
What are the built-in data types in Python?
- Numeric: Integers, floats, booleans, complex numbers.
- Sequence Types:
- Strings
- Lists
- Tuples
- Ranges
- Mapping Types: Dictionaries (key-value pairs).
- Set Types: Unordered collections of unique elements.
5. Mutable vs. Immutable: Understanding Data Type Behavior
What is the difference between mutable and immutable data types?
- Mutable: Can be changed after creation (e.g., lists, dictionaries).
- Immutable: Cannot be changed after creation (e.g., strings, tuples).
Understanding mutability is crucial for avoiding unexpected side effects in your code.
6. Variable Scope: Where Can You Access Variables?
What is variable scope in Python?
- Local: Defined within a function, accessible only within that function.
- Global: Defined outside any function, accessible throughout the program.
- Module-level: Global objects accessible within the current module.
- Outermost: Built-in names that the program can call.
7. Flooring Numbers: The math.floor()
Function
How do you floor a number in Python?
- Use the
math.floor()
function. It returns the largest integer less than or equal to the given number. - Example:
math.floor(3.7)
returns3
.
8. Division Operators: /
vs. //
What is the difference between /
and //
in Python?
/
: Precise division, resulting in a floating-point number (e.g.,5 / 2 = 2.5
).//
: Floor division, resulting in an integer (e.g.,5 // 2 = 2
).
9. For vs. While Loops: Choosing the Right Loop
What's the difference between for and while loops in Python?
- For loop: Iterates through elements of a collection (list, tuple, set, dictionary). Best used when you know the start and end conditions.
- While loop: Executes as long as a condition is true. Best used when you only know the end condition.
10. Passing Functions as Arguments: Higher-Order Functions
Can you pass a function as an argument in Python? Yes!
- Functions are objects and can be passed as arguments to other functions.
- These functions are called higher-order functions.
11. The pass
Statement: A Placeholder
What is a pass
in Python?
- It's a null operation or a placeholder.
- Used when a statement is syntactically required but you don't want to execute any code.
12. break
, continue
, pass
: Control Flow Statements
What are break
, continue
, and pass
in Python?
break
: Terminates the loop or statement.continue
: Skips the current iteration and proceeds to the next.pass
: Does nothing; a placeholder.
13. Argument Passing: By Value or By Reference?
How are arguments passed in Python: by value or by reference?
- Python uses "Pass by Object Reference."
- Immutable objects behave like "pass by value."
- Mutable objects behave like "pass by reference."
14. Lambda Functions: Anonymous and Concise
What is a lambda function?
- An anonymous, small, restricted function.
- Can have any number of arguments but only one expression.
15. Lists vs. Dictionaries: Choosing the Right Collection
How is a dictionary different from a list?
- List: Ordered collection, accessed by index.
- Dictionary: Unordered collection of key-value pairs, accessed by key.
16. List Comprehension: Concise List Creation
What is List Comprehension? Give an Example.
- A concise way to create lists.
- Applies an expression to each item in an existing iterable.
- Example:
[x**2 for x in range(10)]
creates a list of squares from 0 to 9.
17. *args
and **kwargs
: Variable Arguments
What are *args
and **kwargs
?
*args
: Passes a variable number of non-keyword arguments to a function.**kwargs
: Passes a variable number of keyword arguments to a function.
18. Sets vs. Dictionaries: Key Differences
What is the difference between a Set and a Dictionary?
- Set: Unordered collection of unique elements.
- Dictionary: Collection of key-value pairs.
19. Concatenating Lists: Joining Lists Together
How can you concatenate two lists in Python?
- Use the
+
operator or theextend()
method.
20. Docstrings: Documenting Your Code
What is a docstring in Python?
- A string literal that occurs as the first statement in a module, function, class, or method definition. Used to document your code and make it more understandable.
These are just a few of the essential Python interview questions for freshers. Mastering these fundamentals will give you a solid foundation for answering more complex questions and demonstrating your understanding of the language. Good luck, and happy coding!