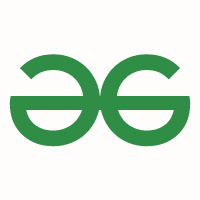
Check String Edit Distance: Simple Guide with C++, Java, Python, C#, and JavaScript Examples
Want to quickly determine if two strings are just one edit away from each other? This guide breaks down the concept of "edit distance" and provides efficient algorithms to check if two strings are within one edit – whether it's adding, deleting, or changing a single character. Plus, we've included ready-to-use code examples in C++, Java, Python, C#, and JavaScript. Let's dive in and simplify your string comparisons!
What is Edit Distance? Understanding Single-Edit String Differences
The edit distance between two strings quantifies how different they are by counting the minimum number of edits required to transform one string into the other. These edits can be:
- Insertion: Adding a character.
- Deletion: Removing a character.
- Substitution: Changing a character.
This article focuses on determining if the edit distance is exactly one, meaning only one of these operations is needed to make the strings identical
Why Check for an Edit Distance of One? Practical Applications
Checking for a single edit distance has several practical applications:
- Spell Checkers: Suggesting corrections for misspelled words.
- Data Cleaning: Identifying and correcting minor errors in datasets.
- Bioinformatics: Analyzing DNA sequences with slight variations.
- Search Algorithms: Improving search results by considering near-matches.
The Efficient Algorithm: How to Check Edit Distance of One
Instead of using complex dynamic programming, we can efficiently check for an edit distance of one by traversing both strings simultaneously. Here's the breakdown:
-
Length Difference: If the absolute difference in lengths of the two strings is more than 1, they can't be at an edit distance of one. Return
false
. -
Initialize: Set an
edit_count
to 0 and use pointersi
andj
to traversestring1
andstring2
respectively. -
Simultaneous Traversal: Iterate through the strings while
i
is less than the length ofstring1
ANDj
is less than the length ofstring2
.-
Mismatch: If characters at
string1[i]
andstring2[j]
don't match:- Increment
edit_count
. - If
edit_count
exceeds 1, returnfalse
. - If
string1
is longer, incrementi
(simulating a deletion fromstring1
). - Else if
string2
is longer, incrementj
(simulating an insertion instring1
). - Otherwise, increment both
i
andj
(simulating a substitution).
- Increment
-
Match: If characters match, increment both
i
andj
.
-
-
Handle Remaining Characters: After the loop, if there are any remaining characters in either string, increment
edit_count
. -
Final Check: Return
true
ifedit_count
is exactly 1; otherwise, returnfalse
.
Code Implementation in Multiple Languages
Below are implementations of the algorithm in C++, Java, Python, C#, and JavaScript:
C++ Implementation
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
bool isEditDistanceOne(string s1, string s2) {
int m = s1.length(), n = s2.length();
if (abs(m - n) > 1) return false;
int count = 0;
int i = 0, j = 0;
while (i < m && j < n) {
if (s1[i] != s2[j]) {
if (count == 1) return false;
if (m > n) i++;
else if (m < n) j++;
else { i++; j++; }
count++;
} else {
i++;
j++;
}
}
if (i < m || j < n) count++;
return count == 1;
}
int main() {
string s1 = "gfg";
string s2 = "gf";
if(isEditDistanceOne(s1, s2))
cout << "Yes" << endl;
else
cout << "No" << endl;
return 0;
}
Java Implementation
Python Implementation
C# Implementation
JavaScript Implementation
Summary: Quick and Efficient Edit Distance Checks
This guide offers a straightforward and efficient way to check if two strings are within an edit distance of one, complete with code examples across multiple popular programming languages. This is valuable for various applications including spell checking, data validation, and more. Implement these algorithms to enhance your projects with rapid string comparison capabilities.