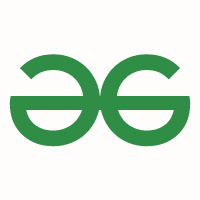
Python String Contains Character? 4 Easy Ways to Check
Do you need to determine if a specific character exists within a Python string? Whether you're validating user input, parsing text data, or just need to perform a quick check, Python provides several efficient methods to accomplish this. This comprehensive guide shows you four simple yet powerful methods to check if a string contains a character using Python.
Why Check if a String Contains a Character?
- Data Validation: Ensure user inputs meet specific criteria (e.g., password complexity).
- Text Parsing: Identify key elements or delimiters in large text files.
- Conditional Logic: Execute different code blocks depending on the presence of a character.
Method 1: Using the in
Operator (Most Pythonic)
The in
operator is the most straightforward and Pythonic way to check for character existence. It returns True
if the character is found and False
otherwise.
- Simple and readable: Offers a clean syntax that's easy to understand.
- Highly efficient: Optimized for membership testing in Python.
Output:
Character found!
Method 2: Leveraging the find()
Method
The find()
method searches for a character within a string and returns its index. If the character isn't found, it returns -1.
- Provides index information: Useful if you need to know the character's location.
- Returns -1 if not found: Makes it easy to implement conditional checks.
Output:
Character found!
Method 3: Utilizing the index()
Method
The index()
method is similar to find()
, but it raises a ValueError
exception if the character is not found.
- Raises an exception when not found: Forces you to handle the "character not present" scenario.
- Good for error handling: Allows you to implement specific actions when a character is missing.
Output:
Character found!
Method 4: Employing the count()
Method
The count()
method counts the number of times a character appears in a string. If the count is greater than 0, the character exists.
- Determines character frequency: Useful if you need to know how many times a character occurs.
- Returns 0 if not found: Simple to integrate into conditional statements.
Output:
Character found!
Choosing the Right Method
- For simple character existence checks, the
in
operator is usually the best choice due to its readability and efficiency. - If you need the character's index, use the
find()
method. - If you want to handle cases where the character is absent through explicit error handling, use
index()
. - If you need to know the frequency of a character, use
count()
.
By understanding these different methods, you can choose the one that best suits your specific needs and write more efficient and maintainable Python code.