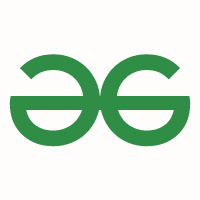
Python Not Operator: The Ultimate Guide to Negating Boolean Logic (with Examples)
Do you want to master the not
operator in Python to write more efficient conditional statements and manipulate boolean values? This comprehensive guide provides practical examples to simplify complex logic, making your code cleaner and easier to understand. Learn how to effectively use the not
operator to invert conditions, check for emptiness, and more.
What is the Python not
Operator?
The not
operator in Python is a logical operator that returns the opposite boolean value of its operand. It's like a simple "on/off" switch for boolean logic. This unary operator takes a single input and flips True
to False
and vice versa. Think of it as the "opposite day" of boolean values!
- The
not
keyword is a unary operator, meaning it works on only one operand. - It is primarily used to reverse the boolean value of an expression or variable.
- Understanding
not
is crucial for controlling program flow and validating conditions.
How to Use the not
Operator?
Using the not
operator is straightforward. Simply place the keyword not
before the expression you want to negate.
Here, the not
operator flips the value of a
from True
to False
. Easy, right?
Practical Applications of the not
Operator
The not
operator is more than just a simple flipping tool. Here's where it shines:
- Boolean Value Manipulation: Primarily used to change
True
toFalse
and vice versa. - Conditional Statements: Used to negate conditions in
if
statements for complex logic. - Data Validation: Checking if a value is not in a collection using the
in
keyword.
Examples of not
Operator in Action
Let's dive into specific examples to see how the not
operator works in different scenarios.
Negating Variables
The most basic use is to negate a boolean variable:
This shows how not
directly inverts the boolean value stored in a variable.
Condition Inversion
The not
operator can be used to change the output of specific conditions.
In each case, the not
operator flips the truthiness of the expression, providing the opposite result.
Handling Different Data Types
The not
operator doesn't just work with booleans. It can also be used with other data types:
Empty strings, lists, and dictionaries are considered False
, while non-empty ones are True
. The not
operator negates these "truthy" or "falsy" values.
Logical NOT with Lists
Here’s an example using the not
operator with lists and conditional statements:
Output:
5 is not in range
10 in range
20 is not in range
59 is not multiple of 5
83 is not multiple of 5
This code snippet uses not
to check whether a list is empty and whether elements meet certain criteria.
Simplify Your Code with not
The not
operator is a small but powerful tool in Python. By understanding how to use it effectively, you can write cleaner, more readable code that expresses complex logic in a straightforward manner. Embrace the not
operator and watch your Python skills grow!