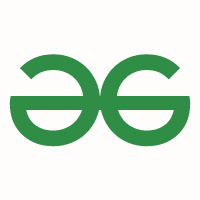
Master the Python List remove() Method: Clear Examples for Efficient Coding
The remove()
method in Python lists is your go-to tool for eliminating specific items. This guide provides a clear, concise breakdown of how to use this method effectively, avoid common pitfalls, and keep your code running smoothly.
What is the Python List remove()
Method?
The remove()
method in Python lists searches the list for the first occurrence of a specified value and removes it. It directly modifies the original list. It’s essential for data manipulation and cleaning within your Python programs.
- Locates and removes the first instance of a given element.
- Modifies the list in place.
- Raises a
ValueError
if the element is not found.
Syntax Explained: How to Use list.remove(obj)
The syntax for utilizing the remove()
method is straightforward. You call it on a list object, passing the element you want to remove as an argument.
list_name.remove(obj)
list_name
refers to the list from which you want to remove an element.obj
represents the object (element) you intend to remove from the list.
Practical Examples of the remove()
Method
Simple Removal
Let's start with a simple example. See how remove()
works in action.
Output:
['a', 'c']
The code snippet removes the first occurence of "b"
in the list a
.
Removing Multiple Elements
The remove()
method can not efficiently remove multiple elements specified as a list. To achieve this, iterate through the list of elements to remove and call remove()
for each.
Output:
[1, 3, 5, 7, 8]
This code iterates through b
, removing each element from a
if it exists. This ensures multiple specific elements are removed.
Handling ValueError
Exceptions
When you attempt to remove an element that isn't in the list, Python raises a ValueError
. You can handle this using a try-except
block.
Output:
Element not found in the list
This approach gracefully handles the scenario where the target element doesn't exist, preventing your program from crashing.
Common Pitfalls and How to Avoid Them
ValueError
: Always check if the element exists before attempting to remove it, or use atry-except
block.- Removing Multiple Occurrences:
remove()
only removes the first occurrence. Use loops or list comprehensions for removing all instances. - Modifying List During Iteration: Avoid modifying a list while iterating over it, as this can lead to unexpected behavior. Use a copy of the list for iteration, or a list comprehension to create a new list.
remove()
Method FAQs
What does the remove()
method do in Python?
The remove()
method removes the first occurrence of the specified element from the list.
Can remove()
remove multiple occurrences of the same element?
No, remove()
only removes the first occurrence of the specified element. Use a loop or list comprehension to remove all occurrences.
Can remove()
be used with an index?
No, remove()
is used with values, not indexes. To remove an element by index, use the pop()
method.
Does the remove()
method return any value?
No, the remove()
method does not return any value. It modifies the list in place and returns None
.