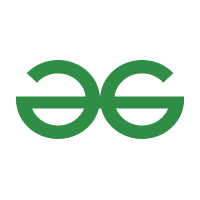
Mastering Python: A Guide to Operators for Sets and Dictionaries
Ready to dive deeper into Python's Sets and Dictionaries? This guide explains the operators that make these data structures incredibly powerful. We'll explore practical examples to solidify your understanding.
Understanding Python Sets Operators
Sets are unordered collections of unique elements. Let's explore the operators that work with them.
Creating Sets: The Basics
Sets are defined using curly braces {}
and elements are separated by commas. Duplicates are automatically removed.
Why Use Frozensets?
Frozensets are immutable versions of sets. Useful when you need a set that cannot be changed after creation, like as a key in a dictionary.
Membership Testing with in
and not in
Quickly check if an element exists within a set.
Comparing Sets: Equality and Inequality
Check if two sets contain the same elements.
Subset and Superset Relationships
Determine if one set contains all elements of another.
s1 <= s2
:s1
is a subset ofs2
.s1 < s2
:s1
is a proper subset ofs2
.s1 >= s2
:s1
is a superset ofs2
.s1 > s2
:s1
is a proper superset ofs2
.
Combining Sets: Union, Intersection, Difference, and Symmetric Difference
Perform set operations to create new sets based on existing ones.
s1 | s2
: Union (elements in either set).s1 & s2
: Intersection (elements in both sets).s1 - s2
: Difference (elements ins1
but not ins2
).s1 ^ s2
: Symmetric Difference (elements in one set or the other, but not both).
Dictionary Operators in Python
Dictionaries store data in key-value pairs. Learn how to manipulate them.
Creating Python Dictionaries
Dictionaries are defined using curly braces {}
and keys are separated from values by a colon :
.
Accessing, Modifying, and Deleting Dictionary Elements
Use these operators to manage key-value pairs.
d[key]
: Get the value associated withkey
.d[key] = value
: Set (or reset) the value associated withkey
.del d[key]
: Delete thekey
and its associated value.
Checking Key Existence
Determine if a key is present in a dictionary.
key in d
: ReturnsTrue
if thekey
is in the dictionary.key not in d
: ReturnsTrue
if thekey
is not in the dictionary.
Comparing Dictionaries
Check if two dictionaries have the same key-value pairs.
Limitations: Subsets and Supersets
The concept of subsets and supersets doesn't apply to dictionaries because they are unordered collections of key-value pairs.
By understanding and utilizing these operators, you can effectively work with Python sets and dictionaries, making your code more efficient and readable. Remember to practice these operations to master them.