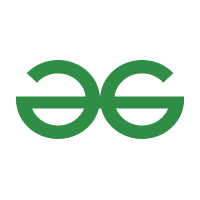
Python: Easily Convert 'YYYY-MM-DD' Strings to Datetime Objects
Working with dates and times is crucial in many programming tasks. Python's datetime
module offers powerful tools for handling date and time operations. This article guides you through converting strings in the 'yyyy-mm-dd' format into datetime
objects. Let's dive in!
Why Convert Strings to Datetime Objects?
Dates are often stored as strings. Converting them to datetime
objects allows you to:
- Perform calculations (e.g., find the difference between two dates).
- Format dates for display.
- Compare dates.
- Use dates in database queries.
Three Ways to Convert Strings to Datetime in Python
Here are three different methods you can use to convert strings to datetime
objects. Select the method that best fits your project requirements.
Method 1: Using strptime()
The strptime()
function from the datetime
module parses a string representing a time according to a format.
- It requires you to specify the exact format of the input string.
- Useful when the date string format is consistent.
Output:
2023-07-25
Method 2: Employing dateutil.parser.parse()
The dateutil
library offers a more flexible approach to parsing date strings.
It automatically detects the format of the date string.
- You'll need to install the
dateutil
library:pip install python-dateutil
- Ideal for handling various date string formats without specifying a format code
Output:
2023-07-25 00:00:00
Method 3: Converting a List of Strings by datetime.strptime()
To convert lists of strings to datetime objects using datetime.strptime()
, iterate through the list. This allows efficient conversion of multiple date strings with the same format.
- Useful for processing large amount of data, when the dates are known.
datetime.datetime.strptime()
converts the input stringi
todatetime
object and.date()
extracts the date.
Output:
2021-05-25
2020-05-25
2019-02-15
1999-02-04
Handling ValueError
with try...except
The strptime()
method raises a ValueError
when the input string doesn't match the specified format. Therefore, error handling is important for code that processes date inputs.
- Use try-except blocks
- Handle unexpected date formats gracefully.
Output:
Error: day is out of range for month
Conclusion
Converting date strings into datetime
objects is a fundamental skill in Python. Mastering these methods will allow you to process date-related tasks efficiently, whether it's calculations, formatting, or comparisons. Use strptime()
for consistent formats, dateutil.parser.parse()
for flexibility, and always handle potential ValueError
exceptions.