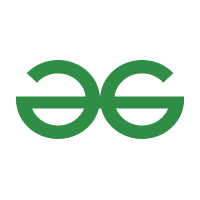
Master String Manipulation in Python: Split and Join Techniques
Are you looking to manipulate strings in Python with ease? This guide dives into the powerful split()
and join()
methods, providing clear examples and practical use cases. Learn how to break down strings and piece them back together, enhancing your Python programming skills.
Why Split and Join Strings in Python?
String manipulation is a fundamental skill in programming. Learning to split and join strings allows you to:
- Parse data: Extract specific information from larger text blocks.
- Format output: Customize the presentation of your data.
- Clean data: Remove unwanted characters or delimiters.
- Build complex strings: Construct new strings from smaller components.
Quick Example: Splitting and Joining
Here's a basic demonstration of how split()
and join()
work:
Output:
Hello,-how-are-you?
Splitting Strings: Breaking It Down
The split()
method is your go-to tool for dividing a string into a list of substrings. Let's explore its variations.
Using string.split()
for Basic Separation
The split()
method, by default, splits a string by whitespace. However, you can specify a delimiter to split the string at specific characters.
Output:
['Geeks', 'for', 'Geeks']
Using splitlines()
for Multi-Line Strings
When dealing with multi-line strings, splitlines()
is incredibly useful. It splits the string at line breaks, creating a list of individual lines.
Output:
['Geeks', 'for', 'Geeks']
Joining Strings: Putting It Back Together
After splitting, you'll often need to combine the pieces back into a single string. The join()
method excels at this.
The Efficient join()
Method
The join()
method is the preferred way to concatenate a list of strings with a specified delimiter. It's efficient and readable.
Output:
Manual Joining with a For Loop (Less Efficient)
While less efficient, using a for
loop to join strings manually provides more control over the joining process. This is useful for applying complex transformations.
Output:
Real-World Applications
- Data parsing: Extracting fields from CSV files.
- URL manipulation: Building or modifying URLs.
- Text processing: Formatting text for display or analysis.
Conclusion
Mastering the split()
and join()
methods in Python will significantly enhance your ability to manipulate strings effectively. Whether you're parsing data, cleaning text, or building complex strings, these tools are essential for any Python programmer.