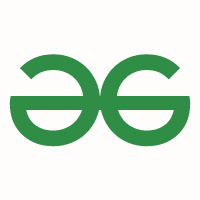
Python List remove()
Method: Clear Examples to Delete Items Effectively
The Python list remove()
method is a powerful tool for modifying lists by element value. It swiftly eliminates the first occurrence of a specified element. This article will guide you through its syntax, usage, and potential pitfalls.
Understanding remove()
Syntax
The syntax is straightforward. Let's break it down:
list_name
: The list you want to modify.obj
: The specific element you want to remove.
Remember that remove()
doesn't return any value. It directly modifies the original list.
Quick Example of remove()
in Action
Here's a simple example showing how remove()
works:
Why remove()
Can Be a Game-Changer
The remove()
method provides a direct way to modify lists based on the value of their items, making your code more readable. It also does not return any value but transforms our list in place, meaning that any other variable referencing our list will be referring to the new list.
Core Concepts Illustrated
-
First Occurrence: remove() only deletes the first instance of the item.
-
In-Place Modification: The original list is directly altered.
-
No return: The method returns
None
.
Common Pitfalls and How to Avoid Them
The Value Error
One common error is attempting to remove an element that doesn't exist, leading to a ValueError
. Let’s see it in action:
Removing Multiple Elements
remove()
isn't ideal when removing multiple occurrences of an element. A loop or list comprehension might be more appropriate
Tackling More Complex Scenarios
Removing elements from a list found in another list
Suppose you have a list of elements to remove from another list, here’s how you can approach this:
The Difference Between remove()
and pop()
It's crucial to understand the difference between remove()
and pop()
:
remove(value)
: Removes the first occurrence of a specific value.pop(index)
: Removes the element at a specific index.
Choose the method that best suits your needs based on whether you know the value or the index of the element you want to remove.
Essential Takeaways
remove()
is excellent for deleting the first instance of a known value.- Use loops or list comprehensions for multiple removals.
- Beware of the
ValueError
if the element is absent—handle withtry...except
blocks. - Understand the distinction between
remove()
(by value) andpop()
(by index).
By mastering these points, you’ll wield the remove()
method with confidence for efficient list manipulation in Python.