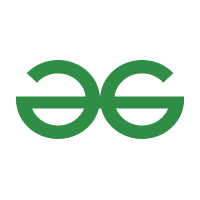
Java Static Methods: The Complete Guide with Examples for Beginners
Struggling to understand static methods in Java? This guide breaks down everything you need to know, complete with clear examples to help you master this essential concept.
What is a Static Method in Java?
A static method belongs to the class itself, not to any specific instance (object) of the class. Think of it as a utility function provided by the class.
- It's declared using the
static
keyword. - You can call it directly using the class name.
- It can access static variables of the class.
Why Use Static Methods? Key Benefits
Why bother with static methods? Here's why they're useful:
- Memory Efficiency: Static methods don't require an object to be created, saving memory.
- Direct Access: Call them directly using the class name, no object needed.
- Utility Functions: Perfect for creating helper functions that operate on class-level data.
Static Method Syntax: How to Declare Them
Here's the basic structure of a static method in Java:
Access_modifier
: Likepublic
,private
, orprotected
.static
: Keyword that defines it as a static method.returnType
: The data type the method returns (e.g.,void
,int
,String
).methodName()
: The name of your method.
Calling Static Methods: Two Simple Ways
You can call a static method in two ways:
Static Methods Can Only Access Static Data: Avoid These Mistakes
One crucial rule: static methods can only directly access static variables (also known as class variables). They can't directly work with instance variables (non-static variables).
- Reason: Static methods exist independently of any object. Instance variables belong to specific objects.
Example 1: Demonstrating Access to Static Variables
This code shows a static method accessing a static variable:
Output:
40
Example 2: Accessing Static Methods Inside Other Methods
Static methods can be called from both static and non-static methods.
Output:
static number is 100
static string is GeeksForGeeks
static number is 100
static string is GeeksForGeeks
Restrictions: What You Can't Do in Static Methods
Keep these limitations in mind:
- No Non-Static Members: Static methods can't directly use non-static variables or call non-static methods.
- No
this
orsuper
:this
(referring to the current object) andsuper
(referring to the parent class) cannot be used within a static method.
Why is Java's main
Method Static? The Real Reason
The main
method is the entry point of every Java program. It's declared as static
so that the JVM can call it directly without creating an object of the class.
- Efficiency: Avoids the overhead of object creation.
- Entry Point: Allows the program to start execution without any instances.
Static vs. Instance Methods: Key Differences
Feature | Instance Methods | Static Methods |
---|---|---|
Object Needed | Requires an object of the class. | Doesn't require an object. |
Access | Can access all attributes. | Can access only static attributes. |
Calling | object.methodName() |
className.methodName() |
Memory | Resides in class object's memory | Stored in the class area. |