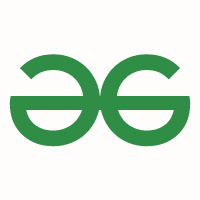
Python String Contains Character? 4 Simple Ways to Check
Want to quickly determine if a Python string contains a specific character? This guide explores four easy methods to achieve this, boosting your Python skills in minutes.
Why Check if a String Contains a Character?
Checking for character presence is fundamental in many tasks such as data validation, text processing, and search algorithms. Learn these methods to write cleaner, more efficient code.
1. The "in" Operator: Python's Easiest Way
The in
operator provides the most straightforward way to check for a character within a string. It returns True
if the character exists, and False
otherwise.
2. find()
Method: Locating Characters by Index
The find()
method searches for a character and returns its index. If the character isn't found, it returns -1
.
find()
is especially useful when you need to know where the character is located in the string.
3. index()
Method: Raising Exceptions for Missing Characters
The index()
method behaves similarly to find()
, but it raises a ValueError
if the character is not found.
Use index()
when you want your program to explicitly handle the case where the character is absent.
4. count()
Method: Counting Occurrences
The count()
method counts the number of times a character appears in a string. If the count is greater than 0
, the character exists.
count()
is perfect when you need to determine the frequency of a character within a string.
Which Method Should You Use?
- For simple existence checks: Use the
in
operator. - To find the character's position: Use
find()
. - To handle missing characters explicitly: Use
index()
. - To count the number of occurrences: Use
count()
.
By mastering these methods, you'll be well-equipped to handle various string manipulation tasks in Python efficiently.