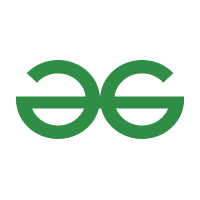
Sort a String of Characters: The Easiest Guide with Examples in C++, Java, Python, C#, and JavaScript
Want to learn how to sort a string of characters quickly and efficiently? This guide provides simple methods with clear code examples in multiple languages. Whether you're prepping for a coding interview or just need a quick solution, we've got you covered!
Why Sort Strings? Understand the Benefits
Sorting strings isn't just an academic exercise. It has practical applications:
- Data Organization: Alphabetize lists and data for easier searching.
- Algorithm Optimization: Some algorithms require sorted input for efficiency.
- Lexicographical Order: Useful in comparing strings and text analysis.
Naive Approach: Sorting with Built-in Functions (O(n log n))
The most straightforward method is to use existing sorting functions in your language of choice. This method is easy to implement but less efficient for very large strings.
C++ Example
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
int main() {
string s = "geeksforgeeks";
sort(s.begin(), s.end());
cout << s << endl; // Output: eeeefggkkorss
return 0;
}
Java Example
Python Example
C# Example
JavaScript Example
Efficient Approach: Counting Sort (O(n))
For strings containing only lowercase characters, we can use counting sort. This method leverages the limited character set to achieve linear time complexity.
How Counting Sort Works
- Count Occurrences: Create an array to store the count of each character (a-z).
- Traverse and Print: Iterate through the count array and print each character the number of times it appears.
C++ Example
#include <iostream>
#include <string>
using namespace std;
const int MAX_CHAR = 26;
void sortString(string& s) {
int charCount[MAX_CHAR] = {0};
for (char c : s) {
charCount[c - 'a']++;
}
for (int i = 0; i < MAX_CHAR; i++) {
for (int j = 0; j < charCount[i]; j++) {
cout << (char)('a' + i);
}
}
cout << endl;
}
int main() {
string s = "geeksforgeeks";
sortString(s); // Output: eeeefggkkorss
return 0;
}
C Example
Choosing the Right Approach
- Built-in Sort: Use for general-purpose sorting or when dealing with a wide range of characters. It’s simple and readily available.
- Counting Sort: Ideal for strings with a limited and known character set (e.g., lowercase letters). It offers better performance (O(n)).
Level Up Your String Sorting Skills
Sorting strings is a fundamental skill that every programmer should master. By understanding these methods and their trade-offs, you'll be well-equipped to handle various string manipulation tasks efficiently.