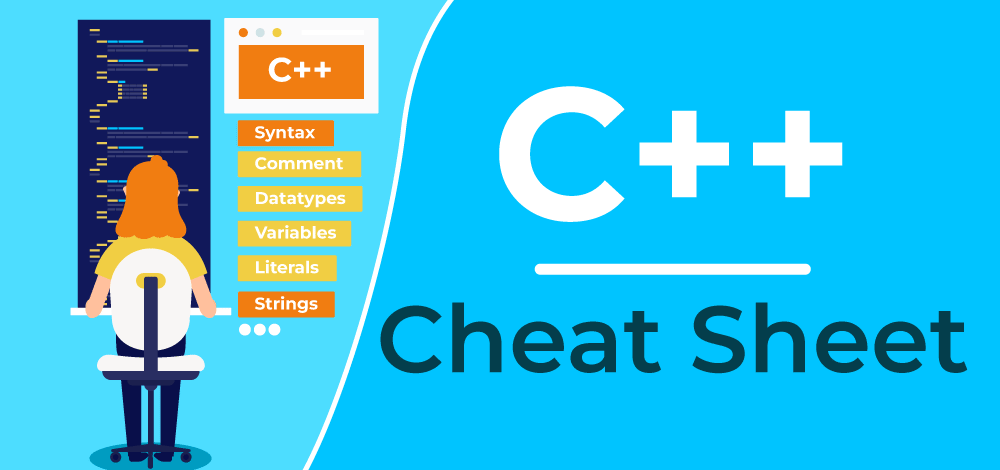
C++ Cheat Sheet: Your Quick Guide to Syntax and Concepts (with Examples)
Are you learning C++ or need a quick refresher? This C++ cheat sheet covers essential concepts, syntax, and examples to help you write efficient and effective code. This guide is great for beginners and experienced coders alike.
C++ is a powerful, high-level programming language used for everything from system software to game development. Let's dive into the fundamentals!
Your First C++ Program: "Hello, World!"
Let's start with a classic example to illustrate the basic structure:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!";
return 0;
}
Output:
Hello World!
Understanding the Basic C++ Syntax
Every C++ program follows a specific structure:
// Header files (Include libraries)
#include <iostream>
// Use standard namespace
using namespace std;
// Main function - execution starts here
int main() {
// Your code statements go here
return 0; // Indicates successful execution
}
#include <iostream>
: Includes the iostream library for input/output operations.using namespace std;
: Avoids repeatedly typingstd::
before elements likecout
andcin
.int main() { ... }
: The main function where the program execution begins.return 0;
: Indicates that the program executed successfully.
Mastering Comments in C++
Comments are essential for code readability and explaining your logic.
- Single-Line Comments: Use
//
to comment out a single line.// This is a comment cout << "GeeksforGeeks";
- Multi-Line Comments: Use
/* ... */
to comment out multiple lines./* This is a multi-line comment. */ cout << "GeeksforGeeks";
Comments are your friends. Use them to explain what you are doing.
Declaring Variables: The Foundation of Storage
Variables are named storage locations for holding data.
- Must be declared before use.
- Can store values of a specific data type.
- Variable names can include letters, digits, and underscores, but must start with a letter or underscore.
Variable Declaration Syntax
// Declaring a single variable
data_type var_name;
// Declaring multiple variables
data_type var1_name, var2_name, var3_name;
Essential Data Types in C++
Data types define the kind of values a variable can hold.
-
Integer (
int
): Stores whole numbers (e.g.,int var = 123;
). Takes 4 bytes of memory. -
Character (
char
): Stores single characters (e.g.,char var = 'a';
). Takes 1 byte of memory. -
Floating Point (
float
): Stores single-precision decimal numbers (e.g.,float num = 1.23;
). Takes 4 bytes of memory. -
Double (
double
): Stores double-precision decimal numbers (e.g.,double num = 1.2345;
). Takes 8 bytes of memory. -
Boolean (
bool
): Stores true or false values (e.g.,bool b = false;
). -
String (
string
): Stores sequences of characters (text). Requires the<string>
header.#include <string> string str = "GeeksforGeeks";
Input and Output Operations in C++
-
Input (
cin
): Reads data from the user.int var; cin >> var;
-
Output (
cout
): Prints data to the console.cout << "Hello World";
-
New Lines: Use
\n
orendl
to insert line breaks.cout << "Hello World!\n"; cout << "Hello World!" << endl;
Conditional Statements: Making Decisions
Conditional statements control the flow of execution based on conditions.
-
if
Statement: Executes a block of code if a condition is true.if (condition) { // Code to execute if condition is true }
-
Nested
if
Statement: Anif
statement inside anotherif
statement.if (condition1) { // Code to execute if condition1 is true if (condition2) { // Code to execute if both condition1 and condition2 are true } }
-
if-else
Statement: Executes one block of code if a condition is true and another if it's false.if (condition) { // Code to execute if condition is true } else { // Code to execute if condition is false }
-
else if
Statement: Checks multiple conditions sequentially.if (condition1) { // Code to execute if condition1 is true } else if (condition2) { // Code to execute if condition1 is false and condition2 is true } else { // Code to execute if all conditions are false }
-
Ternary Operator: A shorthand for
if-else
statements.(condition) ? expression1 : expression2;
-
switch
Statement: Executes different code blocks based on the value of an expression.switch (expression) { case value1: // Code to execute if expression matches value1 break; case value2: // Code to execute if expression matches value2 break; default: // Code to execute if expression doesn't match any case break; }
break
: Exits theswitch
statement.default
: Executed when nocase
matches.
Loops: Repeating Code Blocks
Loops allow you to execute a block of code multiple times.
-
for
Loop: Executes a block of code a fixed number of times.for (initialization; test; update) { // Code to be executed repeatedly }
-
while
Loop: Executes a block of code repeatedly as long as a condition is true.while (condition) { // Code to be executed repeatedly update_condition; }
-
do-while
Loop: Similar towhile
, but executes the code block at least once.do { // Code to be executed repeatedly } while (condition);
Arrays: Storing Collections of Data
Arrays store a fixed-size collection of elements of the same data type.
-
Declaration Syntax:
dataType array_name[size];
-
Example:
#include <iostream> #include <string> using namespace std; int main() { string fruits[] = {"Apple", "Banana", "Orange", "Grapes"}; for (int i = 0; i < 4; i++) { cout << "Fruit at index " << i << ": " << fruits[i] << endl; } return 0; }
-
Multi-Dimensional Arrays
Arrays can have multiple dimensions (e.g., 2D arrays for tables).
-
Declaration Syntax:
data_type array_name[size1][size2]....[sizeN];
Working with Vectors: Dynamic Arrays
Vectors are dynamic arrays that can grow or shrink in size.
- Requires the
<vector>
header.
Vector Declaration Syntax
#include <vector>
vector<data_type> vector_name;
Common Functions
push_back()
: Adds an element to the end.pop_back()
: Removes the last element.clear()
: Removes all elements.empty()
: Checks if the vector is empty.at(i)
: Accesses the element at indexi
.front()
: Accesses the first element.back()
: Accesses the last element.erase()
: Removes an element at a specific position.
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers;
numbers.push_back(10);
numbers.push_back(20);
numbers.push_back(30);
cout << "Element at index 0: " << numbers.at(0) << endl; // Output: 10
return 0;
}
References and Pointers: Working with Memory Addresses
References
A reference is an alias for an existing variable.
-
Declared using the
&
operator.int var = 12; int& ref = var; // ref is a reference to var
Pointers
A pointer stores the memory address of another variable.
-
Declared using the
*
operator. -
Use the
&
operator to get the address of a variable.int i = 3; int *ptr = &i; // ptr stores the address of i
Functions: Reusable Code Blocks
Functions are blocks of code that perform specific tasks.
-
Function Declaration (Prototype): Specifies the function's name, return type, and parameters.
return_type function_name(parameters);
-
Function Definition: Contains the actual code that the function executes.
return_type function_name(parameters) { // Function body return value; // If the function returns a value }
#include <iostream>
using namespace std;
int sum(int a, int b); // Function declaration
int sum(int a, int b) { // Function definition
return a + b;
}
int main() {
int result = sum(3, 4); // Function call
cout << "Sum: " << result << endl; // Output: Sum: 7
return 0;
}
String Manipulation Functions
C++ provides several functions for working with strings.
length()
Function: Returns the length of a string.
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "GeeksforGeeks";
cout << "The length of the string is: " << str.length() << endl;
return 0;
}
substr()
Function: Extracts a substring from a string.
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "GeeksforGeeks";
string sub = str.substr(1, 5);
cout << "Substring: " << sub << endl; // Output: eeksf
return 0;
}
append()
Function: Appends one string to the end of another.
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Geeksfor";
str.append("Geeks");
cout << "Appended string: " << str << endl; // Output: GeeksforGeeks
return 0;
}
compare()
Function: Compares two strings.
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "Geeks";
string str2 = "for";
string str3 = "Geeks";
int result1 = str1.compare(str2);
cout << "Comparison result: " << result1 << endl; // Output: A negative value
return 0;
}
empty()
Function: Checks if a string is empty.
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1 = "GeeksforGeeks";
string str2 = "";
if (str1.empty()) {
cout << "str1 is empty" << endl;
} else {
cout << "str1 is not empty" << endl; // Output: str1 is not empty
}
if (str2.empty()) {
cout << "str2 is empty" << endl; // Output: str2 is empty
} else {
cout << "str2 is not empty" << endl;
}
return 0;
}
Object-Oriented Programming (OOP) Concepts
C++ supports OOP principles.
1. Classes and Objects
A class is a blueprint for creating objects. An object is an instance of a class.
2. Encapsulation
Bundling data (attributes) and methods (functions) that operate on the data within a class.
3. Polymorphism
The ability of an object to take on many forms.
Types of Polymorphism
- Compile-time Polymorphism
- Runtime Polymorphism
4. Inheritance
A mechanism where a new class (derived class) inherits properties and behaviors from an existing class (base class), promoting code reuse and establishing hierarchical relationships between classes.