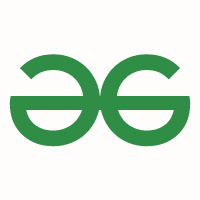
Mastering Python's Ellipsis: A Comprehensive Guide to Three Dots (...)
The ellipsis (three dots: ...
) in Python might seem mysterious at first glance. This comprehensive guide breaks down the ellipsis object, exploring its function and demonstrating practical applications within Python 3. You'll discover how this unassuming symbol plays a crucial role in various coding scenarios.
What is the Ellipsis Object in Python?
The ellipsis (...
) is a built-in Python object, similar to None
. It's a singleton object, meaning there's only ever one instance of it. Crucially, it has no methods of its own. But what does it do? Let's explore its use cases.
Primary Uses of Ellipsis (...
) in Python
The ellipsis serves several distinct purposes:
- As a secondary prompt in the Python interpreter.
- For array slicing and NumPy indexing.
- In type hinting for abstract code.
- As a placeholder or
pass
statement alternative.
Ellipsis as Secondary Prompt in the Python Interpreter
When you're writing multi-line constructs in the interactive Python interpreter, the ellipsis appears as the default secondary prompt.
- It visually indicates that the interpreter expects more input to complete the current statement.
- This is a standard feature for creating functions or conditional blocks directly in the interpreter.
Array Slicing and NumPy Indexing with Ellipsis
Ellipsis shines when working with multi-dimensional arrays, especially with NumPy.
- Accessing: It allows you to select entire dimensions, simplifying indexing.
- Slicing: The ellipsis provides a concise way to slice multi-dimensional data.
Example: NumPy Indexing with Ellipsis
Here’s how to select elements from a 4D NumPy array using ellipsis:
This code snippet demonstrates that [:, :, :, 0]
, […, 0]
and [Ellipsis, 0]
are entirely equivalent ways to select the first element along the last axis.
Important Limitation: You can only have one ellipsis in a single slicing operation. Using multiple ellipsis like array[..., index, ...]
will raise an IndexError
.
Type Hinting with Ellipsis
In type hinting, ellipsis indicates "any" type, offering flexibility.
- Specifies
Any
Type for Function Arguments - Specifies
Any
Type for Function Return Values
Case 1: Any
Type for Arguments
Here, Callable[..., str]
signifies that the function get_next_item
can accept any arguments but must return a string.
Case 2: Any
Type for Return Values
In this scenario, value: ...
suggests that the value
parameter can be of any type, providing flexibility in the function's input.
Ellipsis as a Placeholder (Alternative to pass
)
Ellipsis can take the place of the pass
statement, particularly in function definitions or class structures.
- It signifies that a section of code is intentionally left blank for future implementation.
- Both
pass
and...
achieve the same result, but ellipsis can be more visually distinct.
Using Ellipsis as a Default Argument Value
Ellipsis can be used as a default argument value, especially when you need to differentiate between not passing a value and explicitly passing None
.
This allows you to detect whether an argument was provided or not.
Common Errors When Using Ellipsis
A frequent mistake is using multiple ellipsis in a single slicing operation without colons:
This will result in an IndexError: an index can only have a single ellipsis ('...')
. Always use colons (:
) for accessing other dimensions when using ellipsis.
Conclusion: Mastering the Python Ellipsis
The Python ellipsis is a versatile tool with applications ranging from array manipulation to type hinting. Understanding its purpose and proper usage enhances code clarity and efficiency. By mastering the ellipsis, you add a valuable technique to your Python programming toolkit.