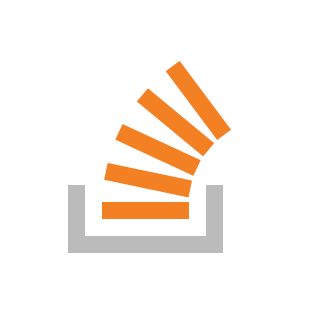
Unlock Compile-Time Magic: Mastering C++ Mapping for Peak Performance
Tired of runtime overhead for command execution? Dive into the world of compile-time map implementations in C++ to achieve blazing-fast performance. Discover techniques for creating efficient, pre-computed mappings, optimizing your code, and leaving runtime maps in the dust!
Why Ditch Runtime Maps for Compile-Time Solutions?
Traditional std::map
incurs runtime costs for lookups. When dealing with fixed sets of commands or configurations known at compile time, pre-computing mappings into a structure yields significant performance boosts. This approach reduces runtime branching, and leverages the compiler's optimization capabilities. Here's why you should make the switch:
- Speed: Lookups become lightning fast using binary search on sorted arrays that are calculated at compile time.
- Efficiency: Minimize runtime computation.
- Constexpr Power: Unleash the power of
constexpr
for supreme performance.
The "Sorted Array" Approach: A Compile-Time Map Alternative
While constexpr std::map
doesn't exist in C++, a sorted std::array
offers an elegant substitute. This strategy creates a pre-sorted array of your commands or keys, enabling efficient binary search at runtime with minimal overhead.
- Ideal for scenarios where the "map" content is known when compiling.
- Leverages sorted data structures for faster searches.
See the following C++ construct showcasing the alternative:
int execute(std::string_view command, int hint)
{
constexpr auto commands = []() {
std::array<std::string_view, 3> temp = {
"cls",
"dir",
"echo",
};
std::sort(temp.begin(), temp.end());
return temp;
}();
static_assert(std::is_sorted(commands.begin(), commands.end()));
enum CommandID
{
#define GET_COMMAND_ID(commandstr) (std::lower_bound(commands.begin(), commands.end(), commandstr) - commands.begin())
cls = GET_COMMAND_ID("cls"),
dir = GET_COMMAND_ID("dir"),
echo= GET_COMMAND_ID("echo")
};
//All of the above will be completed at compile time
int commandID;
if (hint >= 0 && commands.at(hint) == command) {
commandID = hint;
}
else {
commandID = GET_COMMAND_ID(command);
if (commandID == commands.size() || commands[commandID]!= command) {
std::cout << "No matching commands found.\n";
return -1;
}
}
#undef GETCOMMAND_ID
switch (commandID)
{
case cls:
std::cout << "cls\n";
break;
case dir:
std::cout << "dir\n";
break;
case echo:
std::cout << "echo\n";
break;
}
return commandID;
}
This implementation capitalizes on constexpr
to sort the commands at compile time. Subsequent searches within the execute
function then benefit from this pre-computed order.
Compile-Time Map Implementation: Mapping Commands to Functions
For a true compile-time map, you can directly associate string commands with function pointers. This approach completely eliminates runtime map lookups, jumping directly to the correct function based on the input string.
This is how to map commands to functions:
void cls() {
std::cout << "cls\n";
}
void dir() {
std::cout << "dir\n";
}
void echo() {
std::cout << "echo\n";
}
constexpr auto MappingCommandToFunctionPtr(std::string_view command) noexcept{
constexpr auto commands = []() {
std::array<std::pair<std::string_view, void(*)()>, 3> temp = {
std::pair<std::string_view,void(*)()>("cls",cls),
std::pair<std::string_view,void(*)()>("dir",dir),
std::pair<std::string_view,void(*)()>("echo",echo),
};
std::sort(temp.begin(), temp.end());
return temp;
}();
static_assert(std::is_sorted(commands.begin(), commands.end()));
auto temp = std::lower_bound(commands.begin(), commands.end(), std::pair<std::string_view, void(*)()>(command, nullptr), [](const auto& a, const auto& b) {return a.first < b.first;});
return (temp == commands.end() || temp->first!= command)? nullptr: temp->second;
}
This enables direct execution of a function based on the input command string, entirely at compile time.
Key Takeaways for Compile-Time C++ Mapping
- Choose Wisely: Pick your tool based on specific use cases. Sorted arrays are excellent replacements if you don't have
constexpr std::map
; when you want direct function executions, mapping command to function pointers should be considered. - Leverage constexpr: Use
constexpr
whenever possible and practical to push computations to compile time. - Consider Long-Tail Keywords: Explore related terms like "C++ compile time dispatch" or "C++ static map" to find alternative solutions.
- Profile Your Code: Measure the impact of compile-time mapping on your application's performance, making sure it solves the bottlenecks it's supposed to.