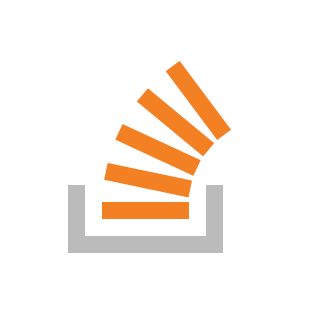
Subclassing Arrays in PeopleSoft: Why Hiding Push() Can Break Your Code
Want to customize array behavior in PeopleSoft PeopleCode by subclassing the Array
class? Hiding the built-in Push
method might seem like a good idea, but it can lead to unforeseen problems. Let's explore why and examine better approaches.
The Pitfalls of Hiding Array Methods
Subclassing and then attempting to hide the Push
method by marking it private sounds like a way to enforce your string-specific logic. However, this directly violates the Liskov Substitution Principle (LSP).
- LSP Defined: LSP dictates that subtypes should be substitutable for their base types without altering the correctness of the program. By hiding
Push
, you might break code expecting standard array behavior.
Understanding the Push
Method Signature
While the PeopleCode documentation mentions Push(paramlist)
, the challenge arises because paramlist
accepts a variable number of arguments. Due to the variable parameters, overriding the signature accurately becomes extremely difficult in some languages.
Composition: A Safer Alternative to Subclassing
Instead of subclassing, consider using composition. This involves wrapping a standard Array
object within your custom class and implementing only the methods you need.
- How Composition Works: Your custom class contains an
Array
object as an instance variable. You then create methods likePushString
to add elements, ensuring they are strings. - Benefits of Composition: This approach avoids the problems associated with inheritance and hiding methods. It also provides greater flexibility and control over your class's behavior.
- Implementation Example: Think of it as building a custom container (string array) that leverages the basic array's functionality without altering its core behavior.
Why "Promotion" is a Misnomer
Calling the action of hiding Push
a "promotion" is inaccurate. You're actually restricting functionality, which goes against the principles of good object-oriented design. Modifying a base method reduces its usability and increases complexity.
Best Practices for Custom Array Handling in PeopleSoft
Instead of trying to manipulate the core Array
class, focus on creating helper functions or wrapper classes that extend its functionality in a non-destructive manner, especially when dealing with specific types such as string arrays. This way, your specialized logic doesn't interfere with code expecting standard array behavior.