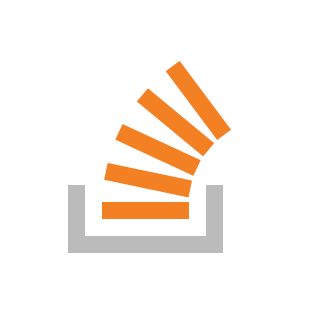
Stack Overflow Hot Topics: Level Up Your Coding Game Now!
Want to stay ahead of the curve in the ever-evolving world of programming? This week's most active questions on Stack Overflow offer a goldmine of insights, solutions, and head-scratchers that every developer should know. Dive in and boost your programming skills! We will also show you how to find Stack Overflow answers more efficiently.
Decoding the Most Popular Questions: What's Trending?
Here's a curated breakdown of the burning questions captivating the Stack Overflow community, designed to help you improve your development workflow and understanding of common coding challenges.
1. File I/O in C: Avoiding Common Pitfalls
The Problem: New C programmers encountering unexpected output when writing and reading files, highlighting the importance of proper file handling techniques.
Key Takeaway: Double-check file opening modes ("r", "w", "a", "r+", "w+", or "a+"), buffer management, and error handling for seamless file I/O. Using the right C programming practices prevents data loss and unexpected behaviors.
2. Dataframe Manipulation in R: Filtering and Cleaning
The Problem: Users seeking efficient methods to filter rows based on conditions and remove irrelevant data in R dataframes.
Key Takeaway: Mastering dplyr and data.table packages allows for fast, readable data manipulation. Learn to use filter()
, slice()
, and regular expressions to extract the data you need and avoid errors. Efficient data analysis with R begins with effective dataframe manipulation techniques.
3. UUID Comparison in Java: Following the Standard
The Problem: Ensuring UUID comparisons adhere to RFC 4122 standards for accurate identification and data integrity.
Key Takeaway: Treat UUIDs as unsigned 128-bit integers and compare them accordingly. Incorrect comparisons can lead to logical errors and security vulnerabilities.
4. Scope of fesetround(): Understanding Floating-Point Rounding
The Problem: Clarifying the scope and impact of fesetround()
on floating-point rounding behavior in C/C++.
Key Takeaway: The scope of fesetround()
is typically thread-specific, affecting floating-point operations within the current thread. Be mindful of its impact when working with multi-threaded applications and ensure consistent rounding behavior when performing complex calculations.
5. C Union and Undefined Behavior: Avoiding Common Mistakes
The Problem: Identifying potential undefined behavior when using C unions, particularly when mixing const
and non-const
members.
Key Takeaway: Be cautious when working with unions, as accessing inactive members can lead to unexpected results. Understand the memory layout and initialization rules to prevent undefined behavior and maintain program stability.
6. Scope Ambiguities in C For Loops: Mastering Variable Declarations
The Problem: Resolving ambiguities regarding the scope of variables declared within the initialization clause of a for
loop.
Key Takeaway: Adhere to coding standards and best practices for variable declaration to prevent scope-related issues. Clear variable declarations are key to writing maintainable code.
7. span<T>
Layout: Understanding Compiler Dependencies
The Problem: Determining whether span<T>
in C++ has a stable layout across different compilers, crucial for inter-compiler compatibility.
Key Takeaway: Passing standard C++ types like span<T>
across compiler boundaries can be risky due to potential layout differences. Consider using a common language subset for better interoperability.
8. Converting std::optional
to std::expected
: Error Handling Strategies
The Problem: Finding the correct approach to convert a std::optional
to a std::expected
, improving error handling in modern C++.
Key Takeaway: Leverage the monadic features of std::optional
along with lambda functions to handle potential errors gracefully during the conversion process, providing cleaner and more robust error handling.
9. Sorting Objects by Multiple Fields: Date and String Comparisons
The Problem: Efficiently sorting a list of objects by a combination of date (as a string) and another string field.
Key Takeaway: Employ custom comparators or lambda expressions to handle the date string parsing and implement a multi-field sorting logic. This technique sorts your data the way you want it. These techniques ensure accurate and flexible sorting based on complex criteria.
10. Removing Suffixes in TSV Files: Data Cleaning with Scripting
The Problem: Removing specific suffixes from columns in a TSV file using scripting languages.
Key Takeaway: Use regular expressions or string manipulation functions in languages like Python or AWK to precisely target and remove the desired suffixes without affecting other parts of the data.
11. Rust Uninitialized Vectors: Avoiding Double Free Errors
The Problem: Understanding the implications of uninitialized memory in Rust and preventing double free errors with vectors.
Key Takeaway: Always initialize vectors properly to avoid undefined behavior and memory corruption. Rust's ownership and borrowing system helps manage memory safely, but understanding the unsafe aspects is crucial for writing robust code.
12. Printing Integers as Floats in C: Format Specifier Errors
The Problem: Understanding why printing an integer with a float format specifier in C results in 0.00.
Key Takeaway: Use the correct format specifiers (%d
for integers, %f
for floats) to avoid unexpected output. Mismatched format specifiers lead to undefined behavior, making debugging challenging.
13. Casting Constants to Structs in C: Understanding Initialization
The Problem: Exploring the ability to cast constants and variables to structs for assignment in C.
Key Takeaway: Understand how C allows for struct initialization through casting. This method can simplify code but requires careful attention to data types and memory layout.
14. Combining Columns with read_table
: Efficient Data Import
The Problem: Combining date and time columns into a single datetime column while using read_table
in data analysis tools.
Key Takeaway: Use the paste()
function in R or similar concatenation methods in other languages to merge the columns during the data import process, streamlining data preparation and analysis.
15. Generating Random Numbers with Constraints: Python Recipes
The Problem: Generating a set of random numbers within a specific range with the constraint that their sum equals a fixed value.
Key Takeaway: Employ techniques like normalizing random numbers or using specialized distributions to meet the sum constraint. Consider Dirichlet distributions for positive numbers and adapt them for signed ranges.
Maximize Your Coding Potential: Actionable Tips
- Stay Curious: Explore these active questions to deepen your understanding of various programming concepts and languages.
- Practice Regularly: Implement the solutions discussed to reinforce your learning and build practical skills.
- Engage with the Community: Participate in Stack Overflow discussions to share your knowledge and learn from others.
- Use Advanced Search Operators: Refine your search using keywords like
[language]
,[library]
,error message
, or specific terms likeperformance
,bug
, andalgorithm
. - Filter by Votes and Answers: Prioritize questions with high upvotes and multiple answers for reliable solutions.
- Check for Duplicates: Before posting, ensure your question hasn't already been answered.
- Example: To find performance tips for Python list comprehensions, search for
"[python] list comprehension performance"
.
By actively engaging with the Stack Overflow community and applying these insights, you'll significantly improve your coding abilities and remain competitive in the tech industry. So, dive in and start learning!