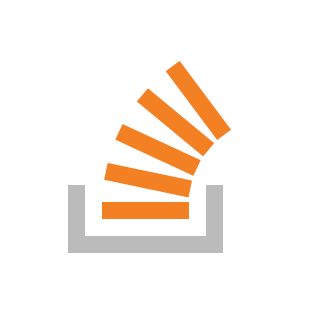
Killer Road Design: Master Bezier Curve Mesh Deformation in Unity
Wish you had smoother, more realistic roads in your Unity game? Learn how to conquer Bezier curve mesh deformation for stunning in-game environments! Ditch those jagged edges and unlock optimized code for peak performance.
The Bezier Curve Mesh Problem: Jagged Edges & How to Slay Them
Creating a road system that bends along a Bezier curve can be challenging. The most common issue involves noticeable seams between segments, resulting in an unrealistic look. Let's troubleshoot this issue.
- The Culprit: Discontinuities in normals and tangents between segments are the main cause.
- The Solution: Smoothing requires a focus on continuity and potentially a smarter approach to mesh construction.
Level Up Your Bezier Curve: Proven Steps to Seamless Meshes
Don't settle for choppy roads. Follow these steps to achieve smooth, seamless transitions in your Bezier road generation workflow.
- Increase Mesh Density Strategically: Instead of uniformly increasing segments, focus density where the curve is sharpest. This balances smoothness with performance.
- Pro-Tip: Use the curve's curvature as a guide for segment density.
- Calculate Normals Per Vertex: Instead of relying solely on face normals, calculate vertex normals by averaging the normals of adjacent faces. This creates a smoother visual appearance.
- Ensure Tangent Continuity: The most crucial step. The tangent vectors at segment endpoints must align. Slight misalignments create the visible seam.
- Your current code calculates tangents using
Vector3.Cross(startDerivative.normalized, Vector3.up)
. Ensure the "up" vector remains consistent and appropriate for all curve orientations.
- Your current code calculates tangents using
- Consider Alternative Mesh Generation: Evaluate smoother mesh generation techniques like:
- Ribbon-based: Generate the mesh as a continuous ribbon following the curve, ensuring tangent continuity.
- Mesh.CombineMeshes: Use multiple smaller meshes and combine them: this provides more flexibility for editing.
Code Optimization: Turbocharge Your Bezier Road System
Your current code has room for improvement. Implement these optimizations during runtime mesh deformation to enhance performance:
- Cache Component References:
GameObject.Find
is expensive. Cache theBezierCreating
component reference inStart()
to avoid repeated lookups. - Precalculate Bezier Points: Calculate and store the Bezier curve points beforehand, especially if the curve doesn't change frequently. This avoids redundant calculations in
Update()
. - Use
StringBuilder
for Debugging: If you're usingDebug.Log
extensively, use aStringBuilder
to construct the message string, improving performance. - Remove Redundant
Clear()
Calls: Clearing thetriangles
list every frame is unnecessary. Clear it only when the number of vertices changes significantly.
Beyond the Basics: Advanced Bezier Road Techniques
Ready to push your skills further? Explore these advanced techniques for creating truly dynamic and immersive road systems:
- Dynamic Road Generation: Generate roads procedurally at runtime based on player input or environment changes.
- Road Texturing: Use advanced texturing techniques like triplanar mapping to create realistic road surfaces that adapt to the curve.
- Deformation Zones: Implement areas where the road can be deformed by external forces like explosions or vehicle impacts.
- Optimize Mesh Colliders: If you need detailed collision, look into creating simplified collision meshes, use convex mesh colliders, or utilize primitive colliders for performance.
Mastering Bezier curve mesh deformation opens doors to creating stunning landscapes and immersive driving experiences in Unity. By implementing these techniques and optimizations, you'll elevate your game development skills and craft truly unforgettable environments.