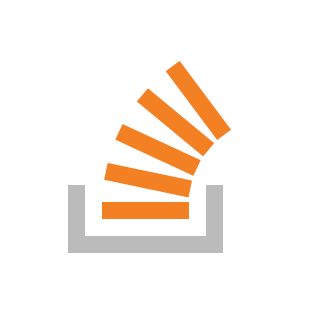
Conquer useSuspenseQuery Testing: A Practical Guide
Struggling to test useSuspenseQuery
in React Query v5? This guide breaks down the common pitfalls and provides actionable solutions to write robust unit tests, covering loading, success, and error states. Let's boost your test coverage!
Understanding the Challenges
useSuspenseQuery
throws a promise when data is loading or an error occurs. This behavior differs from useQuery
, requiring a shift in your testing approach. Directly accessing result.current
during the initial render (while suspending) will likely give you null
because the component hasn't resolved yet.
Scenario Setup: Mocking Made Easy
We use MSW (Mock Service Worker) to simulate API responses. The key is to create separate mock configurations for success, error, and delayed success scenarios:
The QueryWrapperWithSuspense
: Your Testing Ally
This component handles the QueryClient
and the crucial Suspense
boundary. The fallback
prop is essential for testing loading states:
Testing Success: The Happy Path
Here's how to confidently test that data is correctly fetched:
Key Points:
server.use()
: Overrides the global MSW setup for this specific test. This is great when your tests use different API responses.waitFor
&.toBeDefined()
: Wait for theresult.current
to resolve within the suspense boundary, meaning that the Promise thrown byuseSuspenseQuery
has completed.- Timeout: Adjust the timeout in
waitFor
appropriately if your API responses are slow.
Testing Loading States with useSuspenseQuery
The magic is in the fallback
of Suspense
. Check that the fallback is rendered while the hook suspends.
Explanation:
screen.getByText
: Checks if "Loading..." text (set inSuspense
'sfallback
) is present in the DOM before theuseSuspenseQuery
hook resolves.server.use
andctx.delay()
: Configure MSW to return the mocked data after a delay, simulating a loading state.waitFor
: Waits for the component to resolve andresult.current
to be defined.screen.queryByText
: After the data is loaded, we assert that the "Loading..." text is no longer in the DOM, meaning theSuspense
boundary has resolved and the data is displayed.
Testing Errors: Catching the Rejections
When useSuspenseQuery
encounters an error, it throws a promise that rejects. Here's the correct way to test error handling:
Important Points:
await assertThrowsAsync
: BecauseuseSuspenseQuery
throws the rejection, we need toawait
a function that calls therenderHook
statement. React Testing Library's built-inresult.error
reflects the state of the error with theuseSuspenseQuery
hook.result.error
Assertion Verify if theresult.error
(or the error provided by your API) is what's expected. A good implementation can narrow down the type of error and display customized error messages.
Key Takeaways for useSuspenseQuery
- Embrace
Suspense
: TestinguseSuspenseQuery
fundamentally relies on understanding and utilizing theSuspense
component. waitFor
is your friend: UsewaitFor
to ensure your assertions run after the promise resolves (or rejects).- MSW for Control: MSW gives you precise control over your mock API responses, allowing you to simulate various scenarios.
- Check Loading states with
screen
: Usescreen.getByText
andscreen.queryByText
from@testing-library/dom
to make assertions on the Loading states when usingSuspense
.
By following these guidelines, you will develop more reliable and effective tests for components using useSuspenseQuery
, leading to more robust React applications and better user experiences.