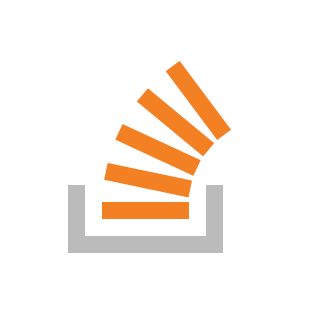
Master Dynamic Data Frame Filtering in R: A Comprehensive Guide
Do you need to filter a data frame in R using a dynamic list of criteria? Struggling to translate complex filtering logic into efficient code? This guide provides several robust solutions, empowering you to filter data frames with ease and precision. We'll explore different approaches using base R and the powerful dplyr
package, ensuring you find the perfect method for your needs.
The Challenge: Filtering with Dynamic Criteria
Imagine you have a dataset like mtcars
and a filter list that changes based on user input or analysis requirements:
filter_list = list(
filter_1 = list(vs = c(0), carb = c(1,4)),
filter_2 = list(cyl = c(4,6))
)
The goal is to filter the data frame so that each element of the filter_list
is evaluated as an "OR" condition, while items within an element are evaluated as "AND". This means we want rows where (vs == 0 AND carb %in% c(1,4))
OR (cyl %in% c(4,6))
. Let's dive into the solutions!
Solution 1: Unleashing dplyr
Power
The dplyr
package provides an elegant and readable way to achieve this data frame filtering. The core idea is to:
- Convert the
filter_list
into a format suitable for joining. - Perform inner joins to filter based on each element of the list.
- Combine the results using
bind_rows()
and remove duplicates.
Here's the code:
library(dplyr)
library(tibble) # Make sure tibble is loaded to use rownames_to_column
# Convert rownames to a regular column
mtcars <- rownames_to_column(mtcars, "car")
filtered_mtcars <- lapply(filter_list, \(filter) expand.grid(filter) %>%
inner_join(mtcars, by = names(filter))) %>%
bind_rows() %>%
distinct(car, .keep_all = TRUE)
print(filtered_mtcars)
Key Benefits:
- Readability:
dplyr
's syntax makes the filtering logic clear. - Efficiency:
inner_join
is optimized for fast data filtering. - Flexibility: Easily adaptable to more complex filtering scenarios.
Solution 2: Base R with eval(parse(text=...))
For those who prefer base R, we can construct a filtering expression as a string and then evaluate it using eval(parse(text=...))
. This approach involves:
- Creating a function that dynamically builds the filtering expression.
- Applying this function to each element of
filter_list
. - Combining the results.
fx <- function(data, x) {
expr <- mapply(function(x, y) sprintf('%s %%in%% c(%s)', x, toString(y)), names(x), x) |>
paste(collapse=' & ') |> str2lang()
subset(data, eval(expr))
}
result_list <- lapply(filter_list, fx, data=mtcars)
#If you want to combine them:
combined_result <- do.call(rbind,result_list)
Why it works:
sprintf
creates the individual filtering conditions (e.g.,"vs %in% c(0)"
).paste(collapse=' & ')
combines these conditions with "AND".eval(parse(text=...))
executes the resulting expression to filter the data frame.
Solution 3: Streamlined Base R using merge
Another base R approach leverages the merge
function for a concise solution:
filtered_data <- do.call(rbind, lapply(filter_list, merge, mtcars))
print(filtered_data)
Advantages:
- Conciseness: Achieves the data frame filtering in a single line.
- Readability: Simple and easy to understand.
- Efficiency:
merge
is well-optimized for joining operations.
Choosing the Right Approach
Each solution has its merits:
dplyr
: Best for readability, maintainability, and complex scenarios.eval(parse(text=...))
: useful when you need to create dynamic filtering conditionsmerge
: Ideal for quick and simple filtering tasks in base R.
Elevate Your Data Manipulation Skills
Mastering data frame filtering is crucial for effective data analysis. By understanding these techniques, you can efficiently extract the information you need, paving the way for deeper insights and informed decisions. Choose the method that best suits your coding style, project requirements, and performance expectations.