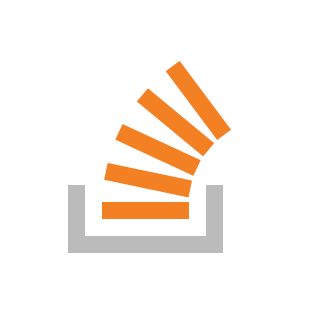
Master C++ Date Manipulation: Find the Next Weekday with Chrono (Boost CTR!)
Do you struggle with date calculations in C++ using the chrono
library? Finding the next or previous weekday can seem complex, but it doesn't have to be. This guide provides clear, concise solutions with practical examples to boost your C++ date manipulation skills. Harness the power of std::chrono
to simplify complex date operations and improve your code's readability and efficiency.
Understanding the Basics: Breaking Down the Problem
Finding the next weekday involves a few key steps:
- Determine the starting weekday: Identify the weekday of your initial date.
- Calculate the difference: Find the number of days between the starting weekday and the target weekday.
- Add the difference: Add that number of days to the initial date.
This approach leverages the std::chrono
library's features to handle the complexities of date arithmetic.
The Efficient next_weekday
Function: Your Code Solution
Here's a highly efficient function to find the next weekday using std::chrono
:
std::chrono::sys_days
next_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
return date + (wd - std::chrono::weekday{date});
}
- This concise function avoids unnecessary temporary variables and leverages implicit conversions for improved performance.
- It directly calculates the difference in days between the current weekday and the target weekday, and then adds that difference to the original date.
- Benefits: Clear, efficient, and easy to understand.
Example Usage:
#include <iostream>
#include <chrono>
int main() {
using namespace std::chrono;
year_month_day date{April/28/2025};
sys_days next_friday = next_weekday(sys_days{date}, Friday);
std::cout << next_friday << '\n'; // Output: 2025-05-02
return 0;
}
Finding the Previous Weekday: A Simple Adjustment
Need to find the previous weekday instead? Just modify the function to subtract the difference:
std::chrono::sys_days
prev_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
return date - (std::chrono::weekday{date} - wd);
}
Example:
#include <iostream>
#include <chrono>
int main() {
using namespace std::chrono;
year_month_day date{April/28/2025};
sys_days previous_friday = prev_weekday(sys_days{date}, Friday);
std::cout << previous_friday << '\n'; // Output: 2025-04-25
return 0;
}
Working with Integers: Converting to and from chrono
Types
Sometimes you start with integer values for year, month, and day. Here's how to convert them to chrono
types and back:
From Integers to chrono
:
int iy = 2025;
int im = 4;
int id = 28;
std::chrono::year_month_day date{std::chrono::year{iy}/im/id}; // Corrected to use {} initialization
From chrono
to Integers:
auto y = date.year(); // year type
auto m = date.month(); // month type
auto d = date.day(); // day type
int iy{static_cast<int>(y)};
unsigned im{static_cast<unsigned int>(m)};
unsigned id{static_cast<unsigned int>(d)};
- Key Point: Use explicit casts to convert
month
andday
to unsigned integers. This ensures proper handling of the underlying data. - Best Practice: While conversion to integers is possible, staying within the
chrono
type system is generally recommended to enable compile-time error checking and improve code robustness.
Handling the Case Where the Input Date is Already the Target Weekday
To get the following weekday, adjust the next_weekday
function to increment the date before the calculation:
std::chrono::sys_days
next_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
date += std::chrono::days{1}; // Increment to get next weekday
return date + (wd - std::chrono::weekday{date});
}
- By incrementing the date before the calculation, you ensure that the function always returns the next occurrence of the target weekday, even if the input date is already that weekday.
Compile-Time Magic: Using constexpr
For even greater efficiency, you can mark the next_weekday
function as constexpr
. This allows it to be evaluated at compile time:
constexpr std::chrono::sys_days
next_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
return date + (wd - std::chrono::weekday{date});
}
static_assert(next_weekday(std::chrono::year_month_day{std::chrono::April/28/2025}, std::chrono::Friday) == std::chrono::year_month_day{std::chrono::May/2/2025});
- Benefits: Faster execution, improved code safety through compile-time checks.
constexpr
ensures that the function can be used in contexts where a compile-time constant is required.
Supercharge Your C++ Date Handling
By understanding these techniques for using the chrono
library, you can efficiently and accurately calculate dates in your C++ applications. These examples provide a solid foundation for handling more complex date manipulation tasks. Master the art of std::chrono
and transform your date-related code from a potential headache into a well-oiled machine.