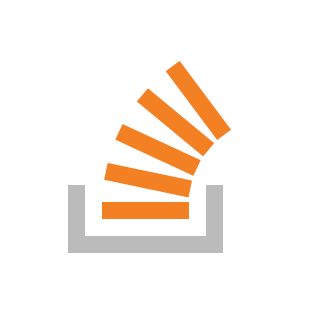
Unlock Cisco Unity Data with VBA: A Practical Guide to REST API Integration (Fixing that 404 Error!)
Are you trying to pull data from your Cisco Unity system into Excel or another VBA-enabled application? This guide will walk you through the process of making HTTP requests to the Cisco Unity REST API using VBA, and, most importantly, troubleshoot that frustrating 404 error some users encounter. By understanding these concepts, you will be well on your path to VBA Unity success.
The Challenge: Accessing Cisco Unity Data Programmatically
Many organizations rely on Cisco Unity for voicemail and unified messaging. Accessing voicemail data programmatically allows for powerful automation, reporting, and integration with other business systems. While Python is a popular choice, sometimes VBA is necessary for users within a Microsoft environment using Excel as their primary tool.
Why VBA for Cisco Unity REST API?
- Integration with Existing Systems: Seamlessly connect Cisco Unity data with Microsoft Office applications like Excel and Access.
- Automation: Automate tasks such as generating reports, analyzing call handler statistics, and synchronizing data.
- User-Friendly Interface: Provide a familiar interface for users who are comfortable with Microsoft Office.
Diagnosing and Fixing the 404 Error: "vmrest/handlers/callhandlers" Not Found
The original poster encountered a 404 error when trying to access the /vmrest/handlers/callhandlers
endpoint. Why does this happen? There are a few likely culprits:
- Incorrect URL: Double-check the URL for typos. Ensure the server IP or hostname is correct. Verify that the
/vmrest/handlers/callhandlers
path is accurate for your Cisco Unity version. - Authentication Issues: The server might be rejecting the request due to authentication failures. Make sure the username and password have permission to access the restful resource.
- API Availability: Confirm that the Cisco Unity REST API is enabled and properly configured on the server.
- Missing Permissions: The user account used for authentication might lack the necessary permissions to access the
/vmrest/handlers/callhandlers
resource. Assign roles like "ApplicationAdmin" to resolve.
VBA Code: A Step-by-Step Guide to Accessing the Cisco Unity REST API using specific call handler ID
This example demonstrates how to retrieve call handler data using a specific ID. Replace the placeholder values with your actual server IP, username, password, and call handler ID.
Sub GetCallHandlerData()
Dim winHttp As Object
Dim strUrl As String
Dim strResponse As String
Dim strUsername As String
Dim strPassword As String
Dim strCallHandlerID As String
' Set your Cisco Unity server IP, username, password, and call handler ID
strUrl = "https://your_unity_server/vmrest/handlers/callhandlers/your_call_handler_id" 'Long tail keyword
strUsername = "your_username"
strPassword = "your_password"
strCallHandlerID = "your_call_handler_id"
' Create WinHTTP object
Set winHttp = CreateObject("WinHTTP.WinHttpRequest.5.1")
' Open the connection with GET method
winHttp.Open "GET", strUrl, False
' Set request headers for authentication
winHttp.SetCredentials strUsername, strPassword, 0
' Send the request
winHttp.send
' Get the response text
strResponse = winHttp.responseText
' Display the response (you can process it as needed)
Debug.Print strResponse
' Clean up
Set winHttp = Nothing
End Sub
Key improvements and explanations:
- Error Handling: While omitted for brevity, robust error handling is crucial in production code to catch unexpected issues.
SetCredentials
: Properly handles authentication by setting credentials directly, preventing issues withstrAuthenticate
.- Data Parsing: While the code retrieves the response, in a real-world scenario, you'd parse the XML or JSON response using VBA's XML or JSON parsing capabilities.
WinHTTP.WinHttpRequest.5.1
: Specifying the version ensures better compatibility.
Actionable Insights for Troubleshooting
- Test with Postman or Insomnia: Use a dedicated API testing tool to verify your credentials and URL are working correctly before implementing in VBA. This helps isolate issues.
- Examine Cisco Unity Logs: Check the Cisco Unity server logs for detailed error messages related to authentication or API access.
- Grant Appropriate Permissions: Ensure your user account has the necessary roles assigned to access resources through the REST API. The "ApplicationAdmin" role is a good starting point.
- Properly Formatted REST API URL: Use the correct address (
https://your_unity_server/vmrest/handlers/callhandlers/your_call_handler_id
) with Call Handler IDs that are valid.
Best Practices for VBA Cisco Unity REST API Integration
- Secure Credentials: Never hardcode passwords directly into your VBA code. Store them securely using Windows Credential Manager or other secure storage mechanisms.
- Error Handling: Implement comprehensive error handling to gracefully handle unexpected errors and provide informative messages to the user.
- Modular Code: Break down your code into smaller, reusable functions for better maintainability.
- Rate Limiting: Be mindful of rate limits imposed by the Cisco Unity REST API. Implement delays or batch requests to avoid being throttled. Understanding VBA rest response limitations helps you design robust integrations.
- XML/JSON Parsing: Learn to use VBA's built-in XML or JSON parsing capabilities (or external libraries) to efficiently extract data from the API responses.
- Consider using long tail keywords in combination for better SEO.
By following these guidelines, you can build robust and reliable VBA integrations with the Cisco Unity REST API, unlocking the power of your unified messaging data.