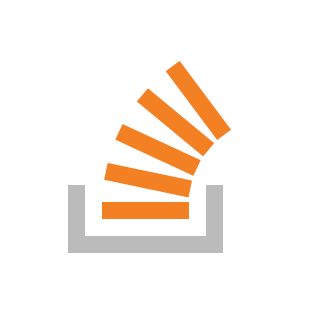
Master Python Mouse Movement: A Beginner's Guide to Precise Control
Struggling to control your mouse with Python? Does your cursor stubbornly refuse to land where you intend it to? You're not alone! Many beginners face challenges when trying to automate mouse actions using Python. This guide will help you tackle common problems and achieve pixel-perfect Python mouse movement using the pynput
library, including using specific mouse coordinates with Python.
Why Your Mouse Might Be Misbehaving (and How to Fix It)
Have you been trying to get the mouse to the right position on the screen but finding it difficult? There are a few reasons why your script may not be working. Here are the common issues when simulating mouse movement, as well as an example of fixing an underlying problem.
- Coordinate System Discrepancies: Your screen's coordinate system might differ from what your script expects.
- Screen Size Issues: Failing to account for your screen size can lead to the script addressing the wrong area in the screen.
If you find you are having problems with your setup script-wise, here is one example of a script with a fix:
pynput
: Your Key to Seamless Mouse Control
pynput
is a powerful Python library designed for controlling input devices. It provides a simple yet effective interface for simulating mouse movement and clicks. Here's a breakdown of how to use it:
- Installation: Install
pynput
using pip:pip install pynput
- Import Necessary Modules: From
pynput
, importmouse
andButton
.
Precise Mouse Coordinates in Python: The Core Functionality
The mouse.Controller()
class within pynput
gives you direct control over the mouse. The key function to using mouse coordinates with Python is the mouse.position
attribute. By setting this attribute to a tuple (x, y)
, you instantly move the mouse to that location on the screen.
Actionable Steps and Best Practices
- Calibrate Your Coordinates: Before running any script, manually use your coordinate-finding script to map key locations on your screen.
- Introduce Delays: Use
time.sleep()
strategically. Adding short pauses between actions allows your system to process commands, preventing errors. - Error Handling: Implement
try...except
blocks to gracefully handle unexpected errors, such as invalid coordinates or library issues.
By implementing the tips above, you'll soon be automating common tasks and controlling your computer with ease!