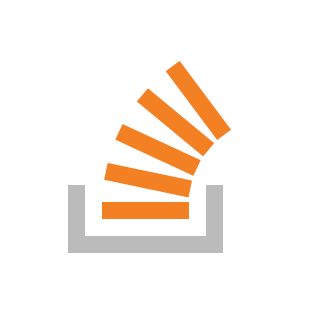
Banishing the Green '+' Bug: Mastering SwiftUI Drag and Drop
That pesky green circle with the plus sign ruining your beautifully designed SwiftUI drag-and-drop interface? You're not alone! Many developers grapple with this visual distraction when implementing object drag behavior, especially when targeting specific drop zones. Let’s dive into how to eliminate it and achieve a polished user experience.
Why is the Green '+' Appearing During Object Drag?
The infamous green "+" icon signals that SwiftUI is attempting to initiate a copy operation during your drag. Even when you intend simply to move the object. This default behavior can clash with your intended design, particularly inside areas where dragging should trigger an action, like moving a swatch to a trash icon. Think of it like SwiftUI trying to be helpful but just getting in the way.
The "presentationDragIndicator" property Might be a Red Herring
While the presentationDragIndicator(.hidden)
modifier sounds like the solution, it isn't always a silver bullet in this scenario. It primarily controls the drag preview appearance, not the core copy behavior indicated by the green "+". This is important to understand because you may be focusing on the wrong element.
The Real Fix: Focusing on Data Transfer and onDrop
The key lies in how SwiftUI handles data transfer during the drag and drop process. Specifically, you need to tell SwiftUI exactly what data you want to transfer and how you want it handled when dropped.
Here’s how:
-
Define a Data Type: Use
NSItemProvider
to encapsulate the data being dragged. This clearly defines the item being moved.- This step dictates how our
object drag behavior
is interpreted by the system.
- This step dictates how our
-
Specify Supported Content Types: In your
onDrag
modifier, specify the supported content types.- Example:
.onDrag { NSItemProvider(object: yourObject as NSString) }
This ensures SwiftUI knows what type of data to expect.
- Example:
-
Handle the Drop with
onDrop
ViewModifier: This is where the magic happens. TheonDrop
modifier gives you control over what happens to the data when it's dropped in the specified area. Inside theonDrop
modifier, theproviders
array gives you an array of transferable data representing the dragged object. For simple cases where you want to receive aString
, you can use this logic:
- Modify the Data Model: If you're moving data you will likely want to remove it from its original location. Make sure you manage the data in a way that you won't have duplicates in your data model.
Example: Dragging a Color Swatch to a Trash Icon
Let's specifically address the color swatch to trash icon scenario.
In this example, we're explicitly telling SwiftUI we're dragging a string, and in our trash target, we process the dropped string representing the color. The green "+" should vanish. Notice that once the item is dropped onto the "Trash" area, it is removed from the colors
array, therefore moving it, instead of copying it.
Mastering onDrop
Parameters
The onDrop
modifier offers parameters for fine-tuning:
of
: Specifies the accepted data types (e.g.,[.text
,.url
,.image]
).isTargeted
: ABinding<Bool>
that indicates when the dragged item is hovering over the drop target. Use this for visual cues.perform
: The closure that executes when the drop occurs, providing an array ofNSItemProvider
objects.
Debugging Tips
- Inspect
providers
: Within youronDrop
closure, print theproviders
array to see what data is being passed. - Content Type Mismatches: Ensure the content types you specify in
onDrag
match the types you expect inonDrop
. - Use breakpoints and step through your code to better understand the flow of execution when dragging and dropping views with SwiftUI's
Object drag behavior
.
By understanding data transfer and leveraging onDrop
effectively, you can eliminate the unwanted "+" icon, creating a smooth and intuitive drag-and-drop experience in your SwiftUI apps. You'll be well on your way to mastering SwiftUI's built in Object drag behavior
.