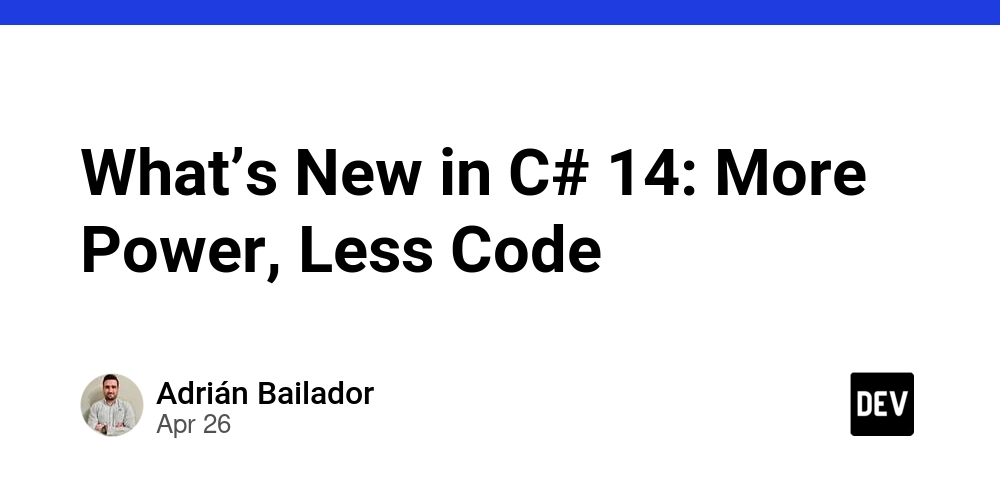
C# 14 Features: Boost Productivity with Shorter, Cleaner Code
.NET developers, rejoice! C# 14 is here, bringing a host of powerful features designed to make your code more expressive, concise, and efficient. This article dives into the core improvements in C# 14, providing clear examples and practical applications to help you get the most out of this upgrade. Let's explore how these updates can significantly improve your coding workflow.
1. Enhanced Extension Members: Add More Functionality to Existing Types
C# 14 significantly expands the capabilities of extension methods. Now you can define properties, indexers, and other members directly within extension blocks. This provides a cleaner, more powerful way to enhance existing types without modifying their original definitions.
- Benefit: Encapsulate reusable logic without altering original type definitions.
- Benefit: Keep your core classes streamlined and focused, while adding functionality only when needed.
2. Streamlined Null-Conditional Assignment in C# 14
The ??=
operator receives a welcome upgrade, extending its functionality to work seamlessly with complex null-conditional expressions.
This enhancement is a game-changer for developers, particularly when handling nested or chained properties that might be null.
- Benefit: Assign values only when a variable is null with cleaner, elegant syntax.
- Benefit: Avoid verbose
if
statements to check for nulls, simplifying your code and enhancing readability.
3. nameof
Operator Extended for Open Generic Types
C# 14 extends the use of the nameof
operator. You can now retrieve the name of an open generic type.
This seemingly small addition offers significant benefits in various scenarios where you need to dynamically reference type names such as in logging frameworks.
- Benefit: Simplifies tasks reliant on string representations of type names with a type-safe method.
- Benefit: Ideal for logging, code generation, and validation purposes.
4. Span and ReadOnlySpan Improvements
C# 14 improves implicit conversions between Span<T>
, ReadOnlySpan<T>
, and arrays (T[]
). This enables safer and easier high-performance memory operations.
- Benefit: Achieve enhanced memory manipulation with implicit conversions.
- Benefit: Facilitate safer, high-performance operations and reduced overhead.
5. Lambda Expressions with Modifiers
C# 14 allows using modifiers like ref
, out
, in
, or scoped
in lambda expressions without explicitly declaring parameter types.
This enhancement simplifies code when working with APIs that involve references or output parameters.
- Benefit: Improves readability by reducing verbosity in lambda expressions interacting with modified parameters.
- Benefit: Simplifies interaction with APIs using references or output parameters.
6. Direct Access to Auto-Property Backing Fields
C# 14 introduces field
, allowing direct access to the backing field of an auto-implemented property.
- Benefit: Enable validation and extended logic directly within property setters without needing to declare a separate private field.
- Benefit: Add validation to auto-properties concisely.
7. Partial Constructors and Events
C# 14 expands partial member support to include constructors and events. This is beneficial in scenarios like code generation or when working with partially generated classes.
- Benefit: Enhances flexibility in code generation and partial class scenarios.
- Benefit: Streamlines modification and extension of generated code segments.