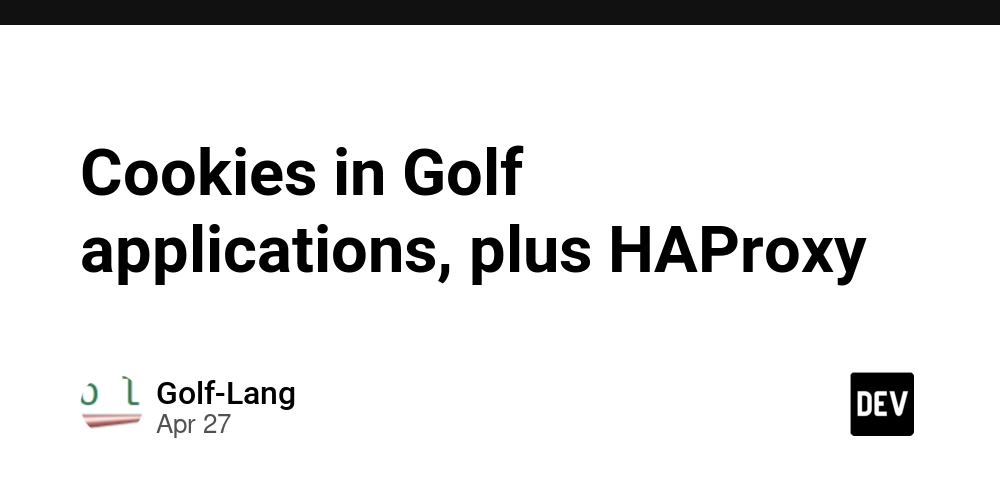
Golf and Cookies: Using HAProxy to Manage User Data Like a Pro
Want to learn how to use cookies in your Golf applications? This guide will show you how to implement cookies for a better user experience and how to pair it with HAProxy. We'll cover a simple example of setting and retrieving a cookie and showcase how HAProxy can be used as a web server or load balancer in conjunction with Golf.
Why Cookies Matter for Your Golf App
Cookies are small text files stored on a user's device by a website. They are essential for:
- Remembering User Preferences: Cookies allow your application to recall user-specific settings.
- Maintaining Session State: They keep track of a user's login status and activity across different pages
- Personalized Experiences: Cookies enable targeted content and advertising based on user behavior.
Set Up Your Cookie-Based Golf App
Let's create a basic Golf application to demonstrate how cookies work. This application will allow users to enter their name, store it in a cookie, and then retrieve it later.
-
Create a New Directory: Start by making a new directory for our "yum" application (because cookies are delicious!).
-
Create the Biscuit.golf Source Code: This code handles the logic for setting and retrieving the cookie.
begin-handler /biscuit get-param action if-true action equal "enter-cookie" // Display a form to get cookie value @<h2>Enter your name</h2> @<form action="<<p-path "/biscuit">>" method="POST"> @ <input type="hidden" name="action" value="save-cookie"> @ <label for="cookie-value">Your name:</label><br/> @ <input type="text" name="cookie-value" value=""><br/> @ <br/> @ <input type="submit" value="Submit"> @</form> else-if action equal "save-cookie" // Submittal of form: save the cookie through response to the browser get-param cookie_value get-time to cookie_expiration year 1 timezone "GMT" set-cookie "customer-name" = cookie_value expires cookie_expiration path "/" @Cookie sent to browser! @<hr/> else-if action equal "query-cookie" // Web request that delivers cookie value back here (to server); display it. get-cookie name="customer-name" @Customer name is <<p-web name>> @<hr/> else-if @Unrecognized action<hr/> end-if end-handler
This code defines different actions based on the "action" URL parameter:
- enter-cookie: Displays a form for the user to enter their name.
- save-cookie: Saves the entered name as a cookie named "customer-name" with a one-year expiration.
- query-cookie: Retrieves and displays the value of the "customer-name" cookie.
Compile and Run Your Golf Application
-
Compile the Application: Make sure all request handlers are public
-
Start the Golf Server: Run the application on port 3000.
Using HAProxy as a Web Server for Your Golf Application
Now, let's configure HAProxy to route requests to our Golf application. This allows us to leverage HAProxy's features like load balancing and security.
-
Configure HAProxy: Modify your HAProxy configuration file (typically
/etc/haproxy/haproxy.cfg
).frontend front_server mode http bind *:90 use_backend backend_servers if { path_reg -i ^.*\/yum\/.*$ } option forwardfor fcgi-app golf-fcgi log-stderr global docroot /var/lib/gg/yum/app path-info ^.+(/yum)(/.+)$ backend backend_servers mode http filter fcgi-app golf-fcgi use-fcgi-app golf-fcgi server s1 127.0.0.1:3000 proto fcgi
- This configuration listens on port 90 and forwards requests starting with
/yum/
to our Golf application. - It also specifies the use of FastCGI protocol for communication with Golf.
- This configuration listens on port 90 and forwards requests starting with
-
Restart HAProxy: Apply the changes by restarting the HAProxy service.
Test Your Setup
Open your web browser and navigate to:
http://127.0.0.1:90/yum/biscuit/action=enter-cookie
You should see the form to enter your name. After submitting the form, you can retrieve the cookie value by visiting:
http://127.0.0.1:90/yum/biscuit/action=query-cookie
Beyond the Basics: Enhancing Your Golf App with Cookies and HAProxy
This example provides a foundation for using cookies in Golf and integrating with HAProxy. You can expand upon this by:
- Implementing more complex cookie management logic.
- Using HAProxy for load balancing across multiple Golf servers.
- Securing your application with HAProxy's security features.
By mastering cookies and leveraging tools like HAProxy, you can create powerful and user-friendly Golf applications. You can also use other web servers like Apache or Nginx, but HAProxy is still a solid choice to use.