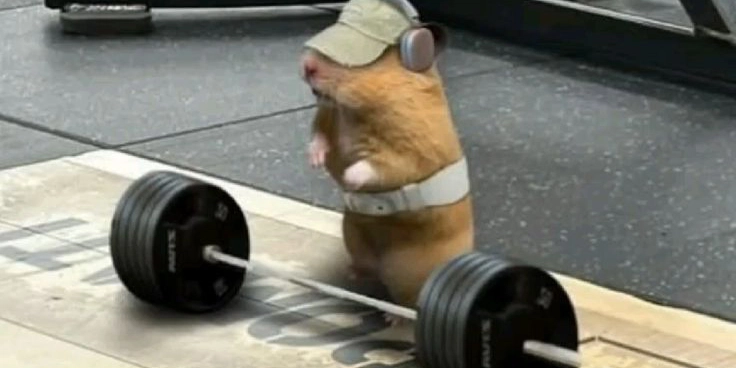
Why C's union
and goto
Aren't Obsolete: A Deep Dive
Are union
and goto
relics of a bygone programming era? Some modern languages are ditching these features, deeming them unsafe or unnecessary. But before you write them off, let's explore why these constructs still hold value in C programming, offering unique benefits and powerful capabilities.
Understanding the Memory Safety Debate
Memory safety has always been a critical concern, leading to the rise of memory-safe languages. While efforts are underway to enhance the safety of C/C++, complete rewrites are often impractical. Projects like TrapC aim to eliminate undefined behavior in C, but at what cost?
The Trade-Off: Performance vs. Safety
Undefined behavior allows C compilers to perform aggressive optimizations. Removing this could impact performance, a key reason why C is chosen for real-time and performance-critical applications. Should we sacrifice speed for safety? Let's delve into why union
and goto
deserve a second look.
union
: Efficient Memory Management and Bitwise Magic
union
allows different data types to share the same memory location. Think of it as a container that can hold multiple things, but only one at a time.
How union
Works: An Example
Consider the following:
Instead of using 8 bytes to store both an int
and a float
, a union
uses only 4, the size of the largest member. union
s shine in scenarios where memory is scarce. They also come in handy for bitwise manipulations, enabling you to reinterpret data in creative ways.
Tagged Unions: Adding Structure & Safety
However, without careful tracking, you lose information about the current data type in a union
. This is where tagged unions come to the rescue. A tagged union combines a union
with an extra field, or tag, to indicate the active data type.
Tagged Unions Enable Type Polymorphism
Tagged unions allow us to create flexible data structures that can hold different types of data, whilst still allowing us to determine what type of data is currently stored. Thus achieving type polymorphism. A feature found in many common languages.
Leveraging Macros for Ergonomics
In C, tagged unions can be verbose. Macros can simplify their use:
This makes creating and using tagged unions much cleaner:
Real-World Applications
Tagged unions are invaluable for:
- Error Handling: Representing results that can either be a value or an error (
Result<T, E>
). - Optional Values: Representing values that may or may not exist (
Option<T>
). - Lexical Analysis: Representing different types of tokens in a compiler (e.g., keywords, identifiers, operators).
goto
: Controlled Jumps for Clarity
The goto
statement allows transferring control to a specific labeled point in the code. Often criticized for creating "spaghetti code," goto
has legitimate uses when applied judiciously.
When goto
Makes Sense
- Error Handling: Centralized error handling becomes cleaner and less repetitive with
goto
. - Breaking Out of Nested Loops: Exiting multiple loops at once is simplified with
goto
.
Here's an example of goto
used for error handling:
goto
in the Linux Kernel
goto
isn't just a theoretical tool. It's prevalent in the Linux kernel for handling cleanup operations. This demonstrates its practical value in complex systems.
Conclusion: Context Matters
union
and goto
are not inherently bad. They're powerful tools that, when used appropriately, can enhance code efficiency and readability. The key is understanding their purpose and applying them strategically.
If you're serious about mastering C, don't let outdated dogma prevent you from exploring the full potential of union
and goto
. They might just be the key to unlocking more ingenious solutions in your programming endeavors.