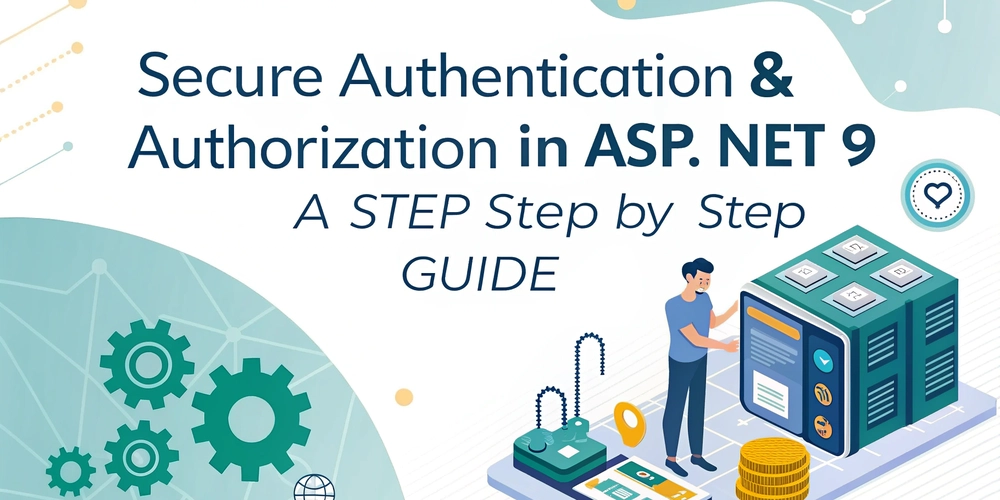
Secure Your ASP.NET Core 9.0 Web Apps: A Practical Guide to Authentication and Authorization
Securing your web applications is a must. This guide dives deep into implementing robust authentication and authorization in ASP.NET Core 9.0. We'll explore the latest features, cover JWT and cookie authentication, and define fine-grained policies to keep your apps protected.
What's Inside?
- Leveraging new authentication/authorization APIs in ASP.NET Core 9.0.
- Step-by-step code examples for JWT and cookie authentication.
- Defining policy- and role-based access to your application resources.
- Applying authentication and authorization to Minimal APIs and MVC controllers.
- Implementing best practices for enhanced application security.
ASP.NET Core 9.0 Authentication: What's New?
ASP.NET Core 9.0 focuses on improved security and a smoother developer experience. Key enhancements include:
- Simplified Configuration: Streamlined extension methods reduce boilerplate code in
Program.cs
. - Built-In Secure Defaults: Automatic enforcement of HTTPS, strict
SameSite
cookie mode, and stricter token validation to enhance security. - Endpoint-Level Authorization: Fine-grained
RequireAuthorization
with policy chaining for Minimal APIs, offering greater control. - Improved DI for Auth Handlers: Custom handlers can directly request scoped services, resulting in more powerful and flexible security policies.
Setting Up Authentication in ASP.NET Core 9.0
Let's jump into the code. Configure authentication services within your Program.cs
before building the application:
JWT Bearer Authentication: Securing Your APIs
JSON Web Tokens (JWT) are commonly used to secure APIs. The following code issues and validates JWT tokens:
Cookie Authentication: Securing Web Applications
Cookies are the traditional approach for authenticating users accessing server-rendered web pages. Example:
Configuring Authorization Policies for Fine-Grained Access Control
Policies define rules that determine user authorization. Register policies in Program.cs
before builder.Build()
:
For more complex authorization logic, use custom authorization handlers:
Securing Minimal APIs and MVC Controllers
After adding app.UseAuthentication()
and app.UseAuthorization()
, secure your endpoints with policy names:.
Minimal API Authorization
MVC Controller Authorization
Best Practices for Secure Authentication and Authorization
Implementing authentication and authorization correctly is essential for application security. Consider these best practices:
- Use HTTPS Everywhere: Enforce proper encryption using
app.UseHttpsRedirection()
. - Short-Lived Tokens & Refresh: Limit the window of exposure if a token is ever compromised.
- Secure Cookie Flags: Always set
HttpOnly
,Secure
, andSameSite=Strict
flags on your cookies. - Validate Inputs & Claims: Never implicitly trust unvalidated data found within claims.
- Centralize Policy Definitions: Keep all your authorization rules consistently defined in a single area.
- Monitor & Log: Track and monitor failed login attempts, token validation issues, and all policy denials.
Conclusion: Secure Your ASP.NET Core 9.0 Applications Today!
This guide demonstrated how to implement secure authentication and authorization in ASP.NET Core 9.0. From setting up JWT and cookie authentication to defining flexible policy-based methods, you're now equipped to build more secure applications. Embrace the latest security features in ASP.NET Core 9.0 to protect your users and data effectively. Feel free to ask questions and provide feedback below!