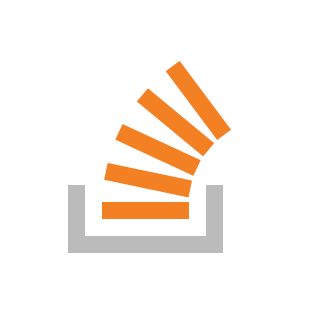
Subclassing Arrays in PeopleSoft: Is Hiding the Push Method a Good Idea?
Want to tailor the Array class in PeopleSoft to handle strings specifically? Many developers consider subclassing, but hiding the Push
method can lead to unexpected issues. Let's explore why and examine alternative approaches.
The Problem: Signature Mismatch and the Liskov Substitution Principle
You're not alone in wanting to restrict functionality in a subclass. The challenge arises when you try to change the Push
method’s visibility PeopleCode complains about a signature mismatch. But why?
The error stems from trying to redefine a parent class method with a different signature, violating the Liskov Substitution Principle (LSP). LSP states that subclasses should be substitutable for their base classes without altering the correctness of the program. Hiding the Push
method breaks this principle, potentially leading to runtime errors if other parts of the PeopleSoft application expect an Array
object to always have a functional Push
method.
Why Hiding Methods in Subclasses is Generally Discouraged
- Breaks Polymorphism: Subclasses should enhance, not restrict, the behavior of their parents. Hiding methods violates this principle.
- Unexpected Behavior: Code relying on the original
Array
class might fail if it encounters your modified subclass. This makes debugging and maintenance difficult. - Potential Conflicts: Future PeopleSoft updates could introduce code that relies on the original
Push
method, leading to conflicts with your subclass.
The Solution: Favor Composition Over Inheritance
Instead of subclassing Array
and trying to hide methods, consider composition.
This is where you wrap the original array in a new class with methods of your choosing.
Here's how composition works
- Create a Wrapper Class: Define a new class, let's call it
StringArrayHandler
. - Embed the Array: Within
StringArrayHandler
, create a private instance of theArray
class. - Implement Desired Methods: Implement methods in
StringArrayHandler
that provide the specific string-handling functionality you need, likePushString
. - Control Access: Only expose the methods you want to be public, effectively "hiding" the original
Push
method of the underlyingArray
object.
Benefits of Composition
- Maintains LSP: You don't modify the original
Array
class, so existing code remains unaffected. - Flexibility: You have complete control over what methods are exposed and how they behave.
- Reduced Risk of Conflicts: Your code is less likely to conflict with future PeopleSoft updates.
For example, the StringArrayHandler
class might include methods to only allow strings into the array, performing validation before adding elements, or maybe all elements should be lower case.
Example: String Array with Case Control
When to Consider Subclassing in PeopleSoft
While composition is generally preferred, subclassing might be appropriate in specific scenarios:
- Adding Functionality: You want to add entirely new methods to the
Array
class without altering its existing behavior. - Extending Behavior: You want to override existing methods to provide specialized behavior, but the subclass must still adhere to the LSP.
Summary: Design Principles for Success
Subclassing the Array
class in PeopleSoft and hiding methods is a risky approach and is most likely not the best solution. Employing composition provides a cleaner, more maintainable solution that adheres to object-oriented design principles. By wrapping the Array
class within a custom class, you can tailor its functionality without breaking existing code or risking future conflicts. Remember to prioritize code clarity and maintainability for long-term success in PeopleSoft development through the proper string array implementation.