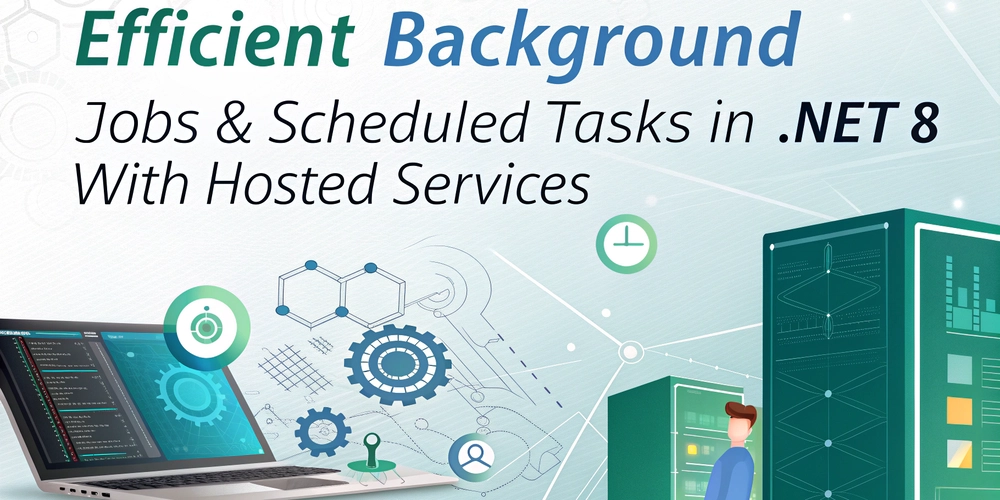
.NET 8 Background Tasks: Mastering Hosted Services for Scheduled Jobs
Want to efficiently run background jobs and scheduled tasks in your .NET 8 applications? This guide explores the power of Hosted Services.
Understanding .NET 8 Background Jobs and Hosted Services
Background tasks are crucial for any .NET application needing to execute processes independent of user interaction. .NET 8's Hosted Services offer a clean, dependency injection-friendly approach for managing such tasks. Forget complex configurations; embrace a streamlined, reliable solution.
Why Use Background Services and Scheduled Tasks?
Background jobs solve critical needs, like:
- Processing message queues without blocking the main application.
- Performing routine data and cache cleanup tasks.
- Delivering emails and notifications reliably.
- Generating reports and aggregating data during off-peak hours.
Hosted Services in .NET 8: Your Background Task Powerhouse
A Hosted Service is simply a class that runs in the background of your application. .NET 8 offers two primary ways to implement them:
- Implementing
IHostedService
: DefineStartAsync(CancellationToken)
andStopAsync(CancellationToken)
methods to control the service's lifecycle. - Deriving from
BackgroundService
: Override theExecuteAsync(CancellationToken)
method for defining long-running loops.
Both methods seamlessly integrate with .NET's Generic Host, offering built-in support for dependency injection, configuration, and graceful shutdown.
Simple .NET 8 BackgroundService Example: Logging Task
Let's see a basic example. This TimedLoggerService
writes a message to the console every 10 seconds:
Register this in your Program.cs
:
.NET 8 Scheduling Recurring Tasks: Timers and Cron Expressions
Using PeriodicTimer
for simple .NET 8 scheduling
Complex Scheduling with Cron Expressions
For more complex scheduling, Cron expressions are your friend! You'll need a library like Cronos:
.NET 8 Handling One-Off Background Jobs with Queues
Sometimes, you need to fire off a task without waiting for it to complete (like sending an email after user registration). This is where a queue comes in handy. Queueing jobs can be very efficient.
Implementing a Background Job Queue
First, define an interface:
Then, implement the queue using a Channel<T>
:
Finally, create a Hosted Service to process jobs from the queue:
Register everything in Program.cs
:
Example : Queueing Email Sending
This shows how you can offload the actual email sending to a background task, improving the responsiveness of your application.
.NET 8 Best Practices for Reliable Background Tasks
- Graceful Shutdown: Always respect the
CancellationToken
to ensure your service shuts down cleanly. - Exception Handling: Wrap your task logic in
try-catch
blocks to prevent unhandled exceptions from crashing your service. - Logging and Metrics: Instrument your service with proper logging and metrics for monitoring and debugging.
- Dependency Injection: Carefully manage the lifetime of injected services.
- Retry Policies: Implement retry logic with exponential backoff for handling transient errors.
- Health Checks: Expose health endpoints to monitor the status of your background services.
Conclusion: .NET 8 Background Processing Mastery
.NET 8 Hosted Services offer a robust and elegant way to manage background jobs and scheduled tasks. By mastering these techniques and following best practices, you can build reliable and efficient applications.