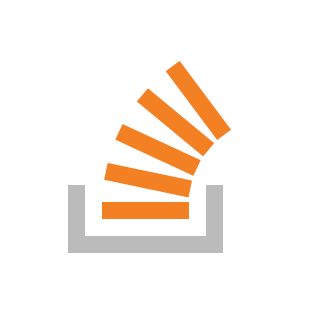
Master C++ Chrono: Effortlessly Find the Next Weekday (and More!)
Are you struggling to manipulate dates and find the next specific weekday in C++ using the chrono
library? You're not alone! This guide breaks down the process into simple, actionable steps, so you can master date calculations and boost your coding efficiency. Learn how to use std::chrono
to find the next weekday, and how to optimize date calculations.
Decoding the Chrono Complexity: Finding the Next Weekday
Let's dive into finding the next weekday using the std::chrono
library in C++. The core idea is to:
- Determine the starting weekday: Figure out what day of the week your initial date falls on.
- Calculate the difference: Determine how many days ahead the target weekday is, compared to the starting weekday.
- Add the difference: Add that number of days to the original date.
Here's the basic C++ code to implement this:
std::chrono::year_month_day
next_weekday(std::chrono::year_month_day date, std::chrono::weekday wd)
{
using namespace std::chrono;
weekday starting_wd{date};
days num_days = wd - starting_wd;
return sys_days{date} + num_days;
}
- This function takes a date and a target weekday as input.
- It calculates the difference in days between the current weekday and the desired weekday.
- Finally, it returns the date of the next occurrence of the specified weekday.
Example:
cout << next_weekday(April/28/2025, Friday) << '\n'; // Output: 2025-05-02
Optimizing for Speed: Using sys_days
While year_month_day
is intuitive, it's not the most efficient for date arithmetic. sys_days
, which represents the number of days since the epoch, is much faster. Here's the optimized version:
std::chrono::sys_days
next_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
std::chrono::weekday starting_wd{date};
auto num_days = wd - starting_wd;
return date + num_days;
}
Benefits:
- Improved performance:
sys_days
avoids unnecessary conversions. - Clean Code: Implicit conversions handle
year_month_day
when needed, adhering to the "pay only for what you use" principle.
You can even compress it into a one-liner:
std::chrono::sys_days
next_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
return date + (wd - std::chrono::weekday{date});
}
Going Back in Time: Finding the Previous Weekday
Need to find the previous weekday instead? Simply subtract instead of add:
std::chrono::sys_days
prev_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
return date - (std::chrono::weekday{date} - wd);
}
Example:
cout << prev_weekday(April/28/2025, Friday) << '\n'; // Output: 2025-04-25
Working with Integers: Converting to and From Chrono Types
Often, you'll have year, month, and day as integers. Here's how to convert them to chrono
types and back:
int iy = 2025;
int im = 4;
int id = 28;
year_month_day next_friday = next_weekday(year{iy}/im/id, Friday);
auto y = next_friday.year();
auto m = next_friday.month();
auto d = next_friday.day();
int iyy{y}; // iyy == 2025
unsigned imm{m}; // imm == 5
unsigned idd{d}; // idd == 2
Key Takeaways:
- Use
.year()
,.month()
, and.day()
to get the parts of the date, these are proper chrono types. - Explicitly cast to
int
orunsigned
when needed. - To convert the other way, construct the chrono types with
year{iy}
,month{im}
, andday{id}
.
Edge Cases: Getting the Next Weekday, Even if It's Today
What if you need the following weekday, even if the input date is already that day? Simple, increment the date before the calculation:
std::chrono::sys_days
next_weekday(std::chrono::sys_days date, std::chrono::weekday wd)
{
date += std::chrono::days{1}; // Use this instead of ++date due to LLVM bug
return date + (wd - std::chrono::weekday{date});
}
Compile-Time Magic: Using constexpr
For ultimate performance, mark your next_weekday
function as constexpr
. This allows the calculation to be performed at compile time!
static_assert(next_weekday(April/28/2025, Friday) == May/2/2025);
Mastering std::chrono
By understanding these core concepts and code snippets, you're well on your way to mastering date manipulation in C++ using the powerful std::chrono
library. Experiment with these techniques and adapt them to your specific needs. You'll be amazed at how easily you can handle complex date calculations!