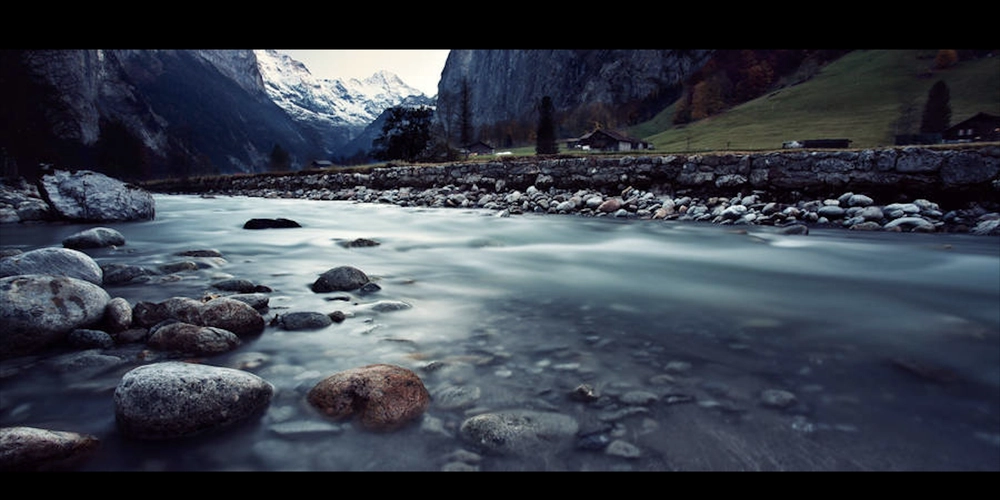
Tame Your React Native Project: How to Master Absolute Imports for Cleaner Code
Tired of getting lost in a maze of ../../../../
in your React Native project? You're not alone. Relative paths can quickly turn into a maintenance nightmare. Absolute imports offer a streamlined, more readable, and ultimately more efficient solution. This guide provides a clear, step-by-step approach to setting up absolute paths in your React Native project.
Why Embrace Absolute Paths in React Native?
Absolute imports provide major advantages over traditional relative paths:
- Clean Code: Say goodbye to endless
../
sequences. Absolute paths make your import statements concise and easy to understand. - Effortless Refactoring: Move files freely without the fear of breaking import statements. React Native absolute paths automatically adjust when files change locations.
- Improved Readability: Instantly understand where components originate, enhancing code comprehension for you and your team.
- Faster Development: Stop wasting time fixing broken paths after refactoring. Utilizing absolute paths minimizes errors and accelerates development.
Step 1: Install the Magic – babel-plugin-module-resolver
This package enables path aliasing, the core of our React Native absolute imports setup. Install it as a development dependency:
Step 2: Configure Your Project for Absolute Harmony
Tell your project where to find your modules by creating a jsconfig.json
or tsconfig.json
file:
For JavaScript Projects (jsconfig.json):
Create a jsconfig.json
file in your project's root directory. Note: If a tsconfig.json
file exists, delete it. The configuration below sets the base URL and defines paths for your components, screens, assets, and navigation.
For TypeScript Projects (tsconfig.json):
Create a tsconfig.json
file in your project's root directory. Ensure that the path aliases match aliases in the babel.config.js
Step 3: Update Babel Configuration
Modify babel.config.js
to include the resolver plugin and define your aliases:
Important: If you aren't using Reanimated, remove the react-native-reanimated/plugin
.
Example: Relative vs. Absolute – A Night and Day Comparison
See the power of absolute paths in action:
❌ The Old (Messy) Way (Relative Paths):
✅ The New (Clean) Way (Absolute Paths):
Troubleshooting: When Things Don't Go as Planned
If VS Code misbehaves:
- Restart Everything: A full restart often resolves indexing issues. Stop the metro server with
Ctrl + C
, close VS Code, remove thenode_modules
folder, and runnpm install
. When done, runnpm start -- --reset-cache
. Reopen VS Code and wait for indexing to complete.
- Verify Configurations: Double-check for typos in
jsconfig.json
(ortsconfig.json
) andbabel.config.js
. Ensure the aliases match and point to the correct directories.
Customization Tips for the Discerning Developer
- Project-Specific Aliases: Replace
app-
with your project's name (e.g.,myproject-components
). - Expand Your Reach: Add aliases for
utils
,services
, orhooks
directories for even cleaner imports. - Team Consistency: Enforce consistent alias usage across your development team.
Final Thoughts: Embrace the Power of Absolute Paths
Switching to absolute paths is a game-changer for React Native projects. The benefits are numerous – cleaner, more maintainable code, easier refactoring, and a smoother development workflow. Implement this simple change and supercharge your React Native development experience!