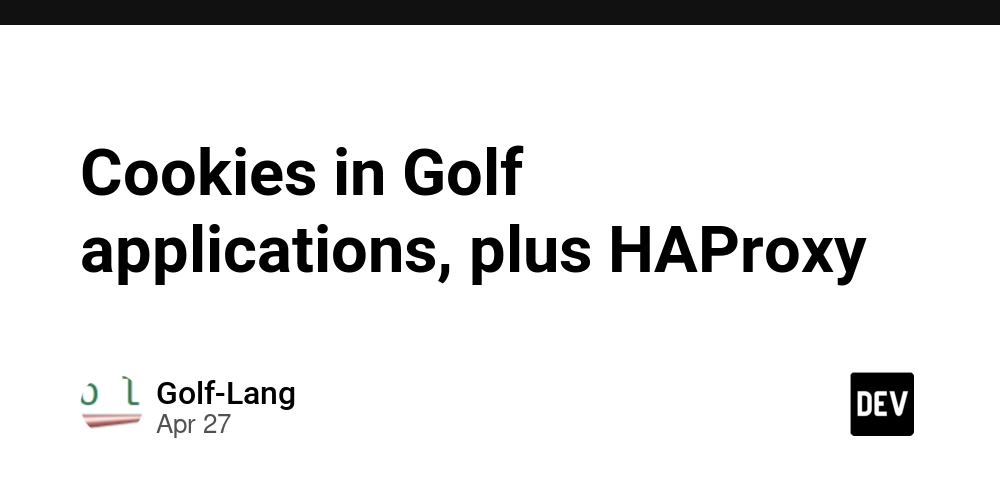
Master Cookies in Golf with HAProxy: A Comprehensive Guide
Cookies are essential for modern web applications, allowing you to store user-specific information and preferences on their devices. This guide dives into using cookies within the Golf programming language, coupled with the powerful HAProxy load balancer. You'll learn how to set, retrieve, and manage cookies, enhancing the user experience and application functionality.
Why Use Cookies in Golf?
Cookies bring several advantages to your Golf applications:
- Personalization: Remember user names, preferences, and settings for a customized experience.
- Session Management: Maintain user sessions, keeping users logged in across multiple pages.
- Tracking: (Ethically) track user behavior and website analytics improving user experience.
Let's explore a practical example to solidify your understanding.
Simple Cookie Handling Example in Golf
Here's a breakdown of a basic Golf application ("yum") that demonstrates setting and retrieving cookies:
1. Create the Application Directory:
2. Create the biscuit.golf
File:
This file contains the Golf code to handle cookie interactions:
begin-handler /biscuit
get-param action
if-true action equal "enter-cookie"
// Display a form to get cookie value
@<h2>Enter your name</h2>
@<form action="<<p-path "/biscuit">>" method="POST">
@ <input type="hidden" name="action" value="save-cookie">
@ <label for="cookie-value">Your name:</label><br/>
@ <input type="text" name="cookie-value" value=""><br/>
@ <br/>
@ <input type="submit" value="Submit">
@</form>
else-if action equal "save-cookie"
// Submittal of form: save the cookie through response to the browser
get-param cookie_value
get-time to cookie_expiration year 1 timezone "GMT"
set-cookie "customer-name" = cookie_value expires cookie_expiration path "/"
@Cookie sent to browser!
@<hr/>
else-if action equal "query-cookie"
// Web request that delivers cookie value back here (to server); display it.
get-cookie name="customer-name"
@Customer name is <<p-web name>>
@<hr/>
else-if
@Unrecognized action<hr/>
end-if
end-handler
Code Explanation
action=enter-cookie
: Displays a form for the user to enter their name.action=save-cookie
: Saves the entered name as a cookie named "customer-name" with a one-year expiration.action=query-cookie
: Retrieves and displays the value of the "customer-name" cookie.
3. Compile the Golf application:
This command compiles, making all request handlers publicly accessible.
Integrating Golf with HAProxy for Enhanced Performance
HAProxy acts as a reverse proxy and load balancer, improving the performance, reliability, and security of Golf applications. Here's how to configure HAProxy:
HAProxy Configuration
frontend front_server
mode http
bind *:90
use_backend backend_servers if { path_reg -i ^.*\/yum\/.*$ }
option forwardfor
fcgi-app golf-fcgi
log-stderr global
docroot /var/lib/gg/yum/app
path-info ^.+(/yum)(/.+)$
backend backend_servers
mode http
filter fcgi-app golf-fcgi
use-fcgi-app golf-fcgi
server s1 127.0.0.1:3000 proto fcgi
Key settings:
bind *:90
: HAProxy listens on port 90.use_backend backend_servers if { path_reg -i ^.*\/yum\/.*$ }
: Routes requests to/yum/
to the Golf application.server s1 127.0.0.1:3000 proto fcgi
: Specifies the Golf server is running onlocalhost:3000
using the FastCGI protocol.
Start your application server
4. Restart HAProxy:
Time to Test!
- Enter a Cookie Value: Open your browser and go to
http://127.0.0.1:90/yum/biscuit/action=enter-cookie
. Enter your name and submit the form. - Retrieve the Cookie: Navigate to
http://127.0.0.1:90/yum/biscuit/action=query-cookie
. You should see your name displayed, retrieved from the cookie.
Beyond HAProxy: Other Web Server Options
While this example used HAProxy, Golf applications can seamlessly integrate with other web servers like Apache and Nginx using FastCGI, providing flexibility in your deployment environment.
Level up your Golf skills!
By following this guide, you've gained practical knowledge of using cookies in Golf and integrating with HAProxy for enhanced performance and scalability. Experiment with different cookie attributes (e.g., secure
, HttpOnly
, and SameSite
) to protect your user data from XSS and CSRF attacks. Use cookies combined with HAProxy effectively, build robust, personalized web experiences.