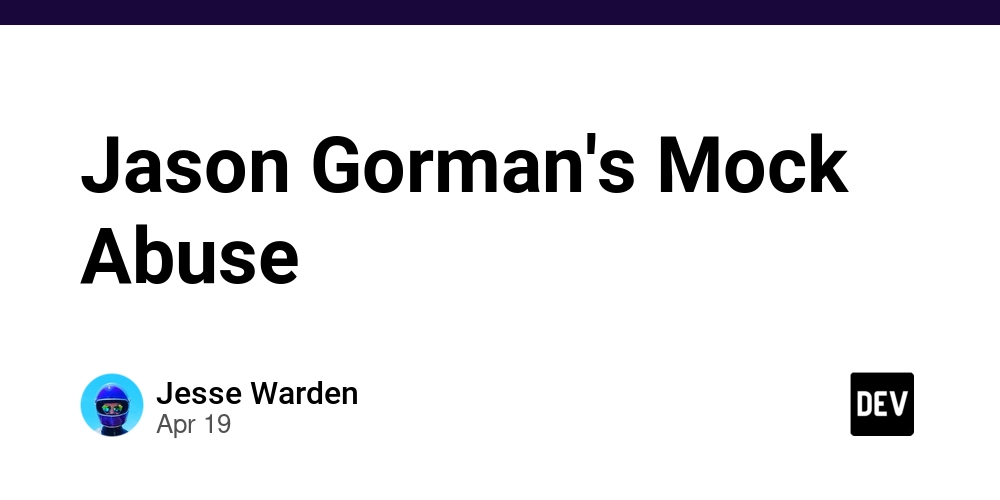
Is Mock Abuse Ruining Your Tests? Spotting the Signs and How to Fix It
Are you spending more time wrestling with your mocks than writing actual code? You might be a victim of "mock abuse," a common problem that leads to brittle tests, code that's hard to refactor, and a general dislike for your test suite. In this article, we'll explore what mock abuse is, why it happens, and how to escape the testing trap.
What is Mock Abuse? Recognizing the "Mock Hell"
Mock abuse, sometimes called "the Mockery" or "Mock Hell," occurs when you overuse mocks in your tests, leading to more problems than solutions. It often indicates deeper design issues within your codebase. Too many mocks are a symptom of a design needing simplification.
- Inability to refactor: The tests are so intertwined with internal implementation that any change breaks them.
- Test suite Loathing: The complexity of maintaining mocks makes testing a dreaded chore.
- Failed behavior validation: Focusing on code coverage metrics instead of verifying real behaviors.
OOP, FP and Dependency Injection
While often associated with Object-Oriented Programming (OOP), mock abuse can also appear in Functional Programming (FP), since both require you to learn, and utilize Dependency Injection. However, it's more prevalent in OOP due to features like interfaces and abstractions. Regardless of the programming paradigm, embracing Dependency Injection is key to writing testable code.
If you’re practicing TDD, then while you’re injecting you’re dependencies, you’ll start asking yourself questions like “Dang, dude… this code requires a lot of dependencies. I don’t like testing this code. Maybe I should change the design?”
Dependency Rejection: Moving Towards Simpler Code
Dependency Rejection helps make as much of the code as pure as possible (it OOP’s case that means classes/methods that have less dependencies, in FP’s case that means more pure functions).
How to Stop Mock Abuse: Design for Testability
The solution to mock abuse lies in designing your code for testability. Here are a few strategies:
- Focus on Single Responsibility: Break down your code into smaller, more focused units that have a single, well-defined purpose.
- Expose a Public API: Design a clear public API for each module or component, and test against that API, testing collaborators together becomes easy as a result. Don't worry about the internal implementation details.
- Embrace "Dependency Rejection": Work towards reducing the number of dependencies each component has, making it easier to test in isolation.
- Visualize Good vs. Bad: Use sequence diagrams to illustrate the difference between well-designed and poorly-designed systems.
Remember, the goal isn't to eliminate mocks entirely, but to use them judiciously. Focus on validating the behavior of your code through its public API, rather than testing internal implementation details. Clean code and proper design patterns help avoid the dreaded "mock hell" and lead to more robust and maintainable tests. Now, grab a beer and refactor!