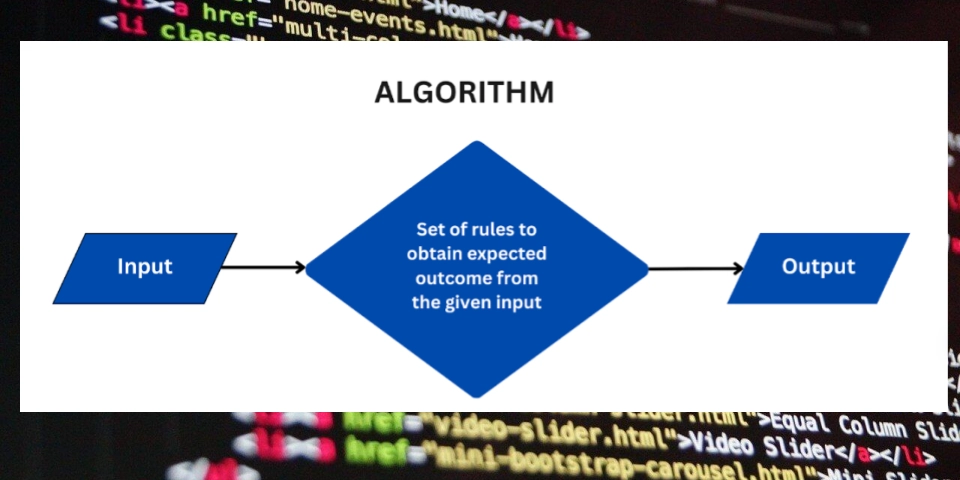
Master JavaScript Algorithms: A Beginner's Guide to Problem Solving
Algorithms are the backbone of efficient and effective code. Are you ready to dive into the world of programming and enhance your problem-solving skills? This beginner-friendly guide breaks down the fundamentals of JavaScript algorithms and walks you through creating your first algorithm.
Unleash Your Coding Potential with Algorithms
Algorithms serve as a roadmap for your code, providing a clear path to solving problems. Think of them as a detailed recipe, providing step-by-step instructions. By understanding and implementing algorithms, you'll write cleaner, faster, and more scalable code.
Why Should You Learn Algorithm Basics?
Learning algorithm basics provides significant benefits, enhancing your skillset and opening doors to new opportunities. Let's explore the key advantages:
- Sharpen Problem-Solving Skills: Algorithms force you to think logically and break down complex problems into manageable steps.
- Write Efficient and Optimized Code: Properly implemented algorithms lead to faster execution and reduced resource consumption.
- Ace Technical Interviews: A solid grasp of algorithms is essential for navigating technical interviews and securing your dream job.
- Build Scalable Applications: Designing applications with algorithms in mind allows them to handle increasing data loads efficiently.
Your First Algorithm in JavaScript: Finding the Maximum Number
Ready to start coding? Here's a simple JavaScript algorithm to find the largest number in an array:
Breaking Down the Code
Let's understand how this simple algorithm works:
- We initially assume the first element of the array is the largest.
- We then create a loop that iterates through each element.
- Every value is compared with current maximum value. If it's greater, we update the maximum with the element.
This basic example demonstrates the core principles of algorithm implementation in JavaScript.
Take Your Learning Further – Comprehensive Video Tutorial
Want a more in-depth understanding with real-world examples and step-by-step coding guidance? Then dive into a comprehensive video tutorial covering:
- A detailed explanation of what algorithms are
- Step-by-step coding examples in JavaScript
- A beginner-friendly approach to complex concepts
- The importance of developing a problem-solving mindset
Watch Introduction to Algorithms with JavaScript (YouTube)
Final Thoughts
Mastering algorithm design with JavaScript requires dedication and consistent practice. However, with a solid foundation and a willingness to learn, you'll be well on your way to crafting efficient, scalable, and high-performing applications. Keep practicing, keep building, and embrace the exciting world of algorithms!