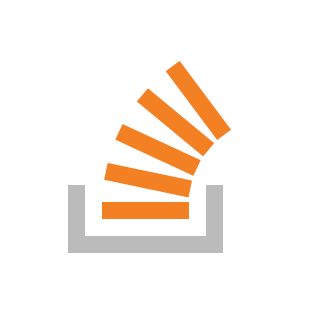
Master Dynamic Dataframe Filtering in R: A Comprehensive Guide
Struggling to filter data.frame using dynamic lists in R? This guide provides several efficient and flexible solutions to tackle this common data manipulation challenge, boosting your data analysis workflow. Learn how to effectively filter data based on complex, dynamically generated criteria.
The Challenge: Filtering DataFrames with Dynamic Lists
Imagine you have a data.frame
and a list of filters. Each element of the list represents an "OR" condition, and each item within that element represents an "AND" condition. How do you apply these filters efficiently?
filter_list = list(
filter_1 = list(vs = c(0), carb = c(1,4)),
filter_2 = list(cyl = c(4,6))
)
The goal is to achieve the same result as the dplyr
code:
library(dplyr)
mtcars %>%
filter(vs %in% c(0) & carb %in% c(1,4) |
cyl %in% c(4,6))
Solution 1: Unleashing the Power of expand.grid()
and dplyr
This method cleverly combines expand.grid()
to create a data frame from the filter conditions with dplyr
's inner_join()
and bind_rows()
for efficient filtering. Let's use expand.grid()
to create a dataframe from nested list.
- Benefit: Leverages
dplyr
's speed and readability for a concise and efficient solution. - How it works:
- Convert row names to a column to preserve them during the join.
- Use
lapply
to iterate through each filter in thefilter_list
. expand.grid
generates all combinations of filter values for each condition.inner_join
filters themtcars
dataset based on these combinations.bind_rows
combines the results from each filter (OR condition).distinct
removes duplicate rows ensuring only unique matches are returned.
library(dplyr)
mtcars <- tibble::rownames_to_column(mtcars, "car") # Preserve row names
lapply(filter_list, \(filter) expand.grid(filter) %>%
inner_join(mtcars, y = .)) %>%
bind_rows() %>%
distinct(car,.keep_all = TRUE)
Solution 2: Crafting Expressions with eval()
This approach dynamically creates R expressions from the filter_list
and evaluates them to filter the data.frame
. Use eval
to create an expression and then eval.
- Benefit: Offers a flexible way to construct complex filter conditions programmatically.
- How it works:
- The function
fx
takes the data and a filter list as input. - It then uses
mapply
to format the filter conditions to be compatible witheval
operation. subset
function then applies the filter.lapply
loops through the filter list.
- The function
fx <- \(data, x) {
expr <- mapply(\(x, y) sprintf('%s %%in%% c(%s)', x, toString(y)), names(x), x) %>%
paste(collapse=' & ') %>% str2lang()
subset(data, eval(expr))
}
lapply(filter_list, fx, data=mtcars)
Solution 3: Concise Base R with lapply
and subset
This elegant base R solution avoids external packages, providing a compact and efficient way to filter. Use lapply
to evaluate parse d strings, where we use collapse='&' in paste().
- Benefit: Pure base R solution, minimizing dependencies.
- How it works:
lapply
iterates through each filter infilter_list
.paste
constructs a character string representing the filter condition (e.g., "vs %in% c(0) & carb %in% c(1,4)").parse(text=...)
converts the string into an R expression.eval
evaluates the expression within thesubset
function to filter themtcars
data.
lapply(filter_list,
\(i) subset(mtcars, subset=eval(parse(text=paste(names(i), '%in%', i, collapse='&')))))
Solution 4: Merging with Base R
This method utilizes the merge
function in base R for a concise solution.
- Benefit: Compact base R solution.
- How it Works:
- Uses
lapply
loop throughfilter_list
. - Applies merge to
mtcars
. - Uses
rbind
to combine the results.
- Uses
do.call(rbind, lapply(filter_list, merge, mtcars))
Key Takeaways for Mastering Dynamic DataFrame Filtering
- Flexibility: These methods adapt to varying filter conditions without modifying the code structure.
- Efficiency:
dplyr
and base R solutions optimize filtering speed. - Readability: Choose the method that balances conciseness and clarity for your coding style.
By mastering these techniques, you'll be well-equipped to tackle any data.frame filtering challenge with dynamic lists, enhancing your data analysis skills significantly. Embrace the power of R and transform your data manipulation workflow today!