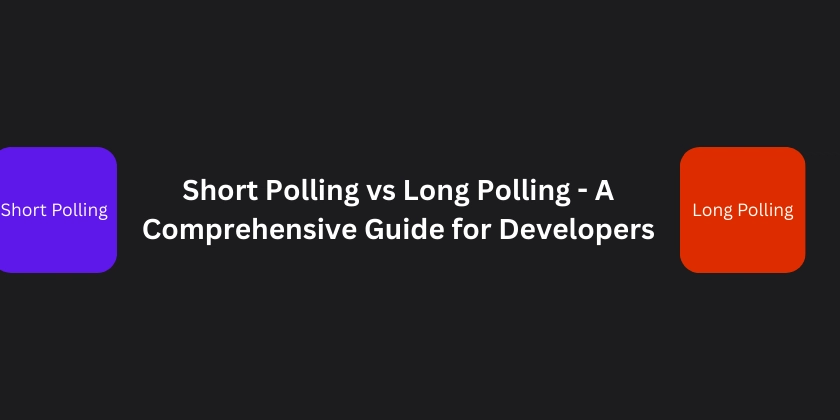
Short Polling vs. Long Polling in React: Choose the Best Data Update Strategy
Want to keep your React UI fresh and updated? You'll likely need to implement a polling technique. Discover the differences between short polling and long polling and learn how to implement them for a dynamic, real-time experience. Let's dive in and determine which data-fetching strategy is right for your next project!
What is Short Polling? The Frequent Checker
Short polling is like a child constantly asking, "Are we there yet?". Your React app repeatedly checks with the server at regular intervals for updates.
Here's what short polling looks like in React code:
Advantages of short polling in React:
- Super straightforward to implement with a simple interval.
Short polling
works even with basic server setups that don't support fancy connection handling.
Disadvantages of short polling:
- Can be wasteful - constantly pinging the server when nothing changes.
- Your server might start sweating under load with many users constantly pinging it, causing performance trouble.
What is Long Polling? The Patient Waiter
Long polling is like leaving your number with a store and asking them to call when your order is ready. Your app asks the server once, and the server waits until it has something new to tell you. It's a more efficient way to get real-time updates
.
Here's how to implement long polling in React
:
Advantages of Long Polling in React
- Much kinder to your server when updates are infrequent, reducing server load.
- Feels snappier to users since updates come through almost immediately.
Disadvantages of Long Polling
- Your server needs to handle holding connections open, which all servers can't do well.
Long polling
requires a bit more care to set up correctly.
Short Polling vs. Long Polling: Which Should You Choose?
Here's a practical guide to making the right choice between short polling and long polling for your React application:
Scenario | Go with... | Because... |
---|---|---|
Updates happen every few minutes | Short Polling | No need to complicate things when simple works. |
A chat app where seconds matter | Long Polling | Users will appreciate the near-instant updates. |
Legacy server that chokes on connections | Short Polling | Work with what you've got! |
Production app that needs to scale | Long Polling | Lower server load as user counts climb. |
Limited control over your backend | Short Polling | No special server-side logic needed. |
Friendly tip: For lightning-fast updates for a multiplayer game or collaborative editor, skip polling altogether and look into WebSockets or Server-Sent Events!
Common Polling Mistakes to Avoid
- Polling too frequently: Be realistic about how "real-time" you need to be to avoid extra server calls.
- Forgetting error handling: Always include proper error handling and retry logic, or your polling will silently stop working when things go wrong.
- Creating zombie processes: Clean up your intervals or recursive polling functions when components unmount.
- Overloading servers with long polling: Make sure your infrastructure is up to the task before implementing long polling at scale.
- Sticking with polling when better options exist: For truly
real-time data
, WebSockets often provide a cleaner, more efficient solution.
Conclusion: Short Polling vs Long Polling
Both techniques have their place in a developer's toolkit:
- Short polling is your reliable solution for simple apps with infrequent updates.
- Long polling delivers that near real-time feel without completely reinventing your architecture.
The best choice ultimately depends on your specific app needs, your server capabilities, and how much you value that real-time experience for your users.