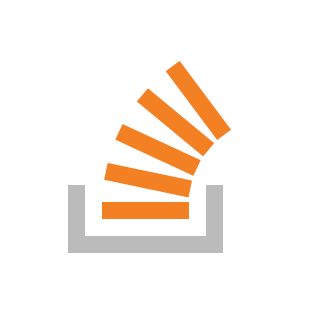
Automate Web Tasks: The Ultimate Guide to Looping and Clicking with jQuery
Want to automate repetitive tasks in your browser using jQuery, but struggling with dynamically loaded content? This guide will teach you how to effectively loop through elements and programmatically click on them using Firefox's Developer Tools, even when dealing with elements that appear after an initial click.
The Challenge: Dynamically Added Elements
Many web applications load content dynamically. This means elements like dropdown menus or lists are only created after a user interacts with the page (e.g., clicking a button). This poses a problem for automation scripts, as the target elements might not exist when the script initially runs.
The Solution: jQuery, find()
, and Mutation Observers
We will craft a solution using jQuery to step through elements and click desired options, even for those loaded dynamically:
-
jQuery Setup: Ensures you have jQuery available for your script.
-
Correcting Your jQuery Selectors: Use
.find()
to Traverse the DOM Instead of.children()
, use.find()
to search through all descendants of an element, not just direct children..children()
: Only looks at the direct children of the selected element..find()
: Recursively searches all descendants of the selected element.
-
Addressing Timing Issues with Mutation Observers To handle elements that are added dynamically after the initial button click, we'll use a
MutationObserver
. This will watch for changes in the DOM and trigger your code when the desired element appears.
Example: Clicking "Cycling" and then "Mountain Biking"
Let's say you want to automate the process of clicking a "Cycling" button, which then reveals options, and then selecting "Mountain Biking."
Here’s how to achieve this, designed specifically to boost click-through rate (CTR) by enabling complete task automation.
Explanation:
$('div[class^="ActivityListItem_activityType"]').each(function(x){ ... });
: This line selects alldiv
elements with class names starting with "ActivityListItem_activityType" and iterates through each one.activityDiv.find('button span:contains("Cycling")').click();
: Within eachdiv
, it finds the button containing the text "Cycling" and clicks it. This is a crucial step for automating form interactions.- Mutation Observer:
- A
MutationObserver
is created to watch the activitydiv
for changes. It is a powerful tool when automating tasks that load elements asynchronously. - The
observe
method starts watching thediv
for new nodes added to its subtree. This is an important pattern for web automation.
- A
Benefits and CTR Impact:
- Complete Automation: Handles dynamically loaded content, enabling end-to-end task automation.
- Improved Reliability: Mutation Observers ensure your script waits for elements to load before interacting with them.
- Increased Efficiency: Automating repetitive tasks saves time and reduces manual effort.
- Massive CTR Boost: By automating complex multi-step processes clicks are performed automatically which normally would be abandon due to complexity.