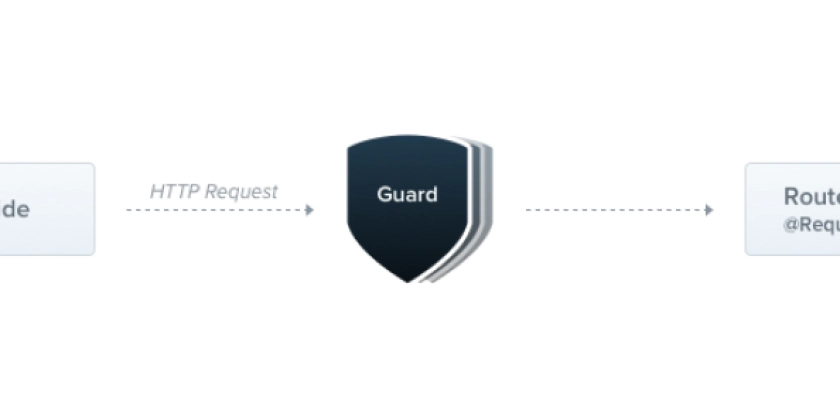
NestJS Guards: Master Testing Strategies for Robust Authorization
Want to ensure your NestJS application's authorization is rock solid? Many NestJS testing articles only scratch the surface. This guide dives deep into effective strategies for testing NestJS Guards, showing you how to write robust, maintainable tests that prevent unwanted access. Learn how to comprehensively test your authorization logic focusing on integration tests, unit tests, and end-to-end (e2e) tests.
TL;DR: Testing NestJS Guards Like a Pro
- Integration Tests First: Simulate real endpoint access using a test controller within a dedicated test module.
- Isolate Logic for Unit Tests: Extract complex authorization logic into separate, testable functions.
- E2E Tests for Key Scenarios: Validate happy paths and crucial "negative" scenarios for guarded endpoints.
WordWiz Example: Subscription-Based Content Generation
Imagine "WordWiz," an AI-powered content generation app. Access to features depends on the user's subscription tier: Free, Basic, or Premium.
Here's how access is structured:
- Free: Limited access, basic AI.
- Basic: Better AI, higher limits.
- Premium: Unlimited content, advanced AI, SEO analysis.
The application exposes three key endpoints:
POST content/generate
: AI content generation (Free+)GET content/templates
: Content templates (Basic+)GET content/analytics
: Engagement insights (Premium+)
We'll use a SubscriptionGuard
and header x-user-subscription
to enforce access based on the user's plan.
Tests CAP Theorem: Balancing Act
Good automated tests have four pillars:
- Protection Against Regressions: How well tests catch bugs.
- Resistance to Refactoring: Tests don't break with internal changes.
- Fast Feedback: Test execution speed.
- Maintainability: Ease of maintaining test code.
Like the CAP theorem, you can't maximize everything. Prioritize resistance to refactoring to avoid brittle tests. Then, choose between fast unit tests or higher protection from e2e tests.
Integration Tests: Seeing the Whole Picture for Testing Guards
Integration tests provide a good balance between protection and feedback speed.
Steps include:
- Send a request to a test endpoint protected by a
SubscriptionGuard
. - The
SubscriptionGuard
either accepts or rejects the request. - The client receives the corresponding success or error response.
Let's consider the subscriptions.guard.spec.ts
:
Breakdown
TestController
: Defines endpoints with different subscription requirements using@RequiresSubscription()
decorator. Register the guard correctly.TestModuleForGuard
: Sets up the testing module, registering theSubscriptionsGuard
as a provider.beforeAll
: Initializes the testing module and starts a local HTTP server.- Test cases: Cover basic assumptions about how the
SubscriptionsGuard
should work by settingSUBSCRIPTION_HEADER
with different subscription plans. Verify success or failure responses accordingly.
Here's a basic implementation for SubscriptionGuard
:
These robust integration tests quickly validate the initial guard implementation.
Unit Testing the Subscription Logic: Humble Object Pattern
For complex logic, apply the Humble Object Pattern: extract the subscription comparison logic and test it in isolation for faster feedback.
Tests defined as follows:
Implementation of business logic is defined as follows:
Update the guard implementation to use the new abstracted function:
Finally, re-run all tests to confirm stable behavior.
E2E Tests: Validating the Real Deal
E2E tests validate the entire structure, ensuring it fulfills the "WordWiz" subscription requirements. Focus on testing the highest subscription requirement for each endpoint and negative scenarios: A free user should not have access if they do not have permissions.
Create E2E tests that validate real endpoints like content/generate
, content/templates
, and content/analytics
work as expected.
Key Takeaways: Solid NestJS Guard Testing
By combining integration, unit, and e2e tests, you can comprehensively validate your NestJS Guards. Start with integration tests, isolate logic for unit tests, and use e2e tests to confirm real-world behavior. This approach ensures robust authorization and a secure application.